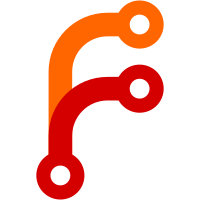
It is not inherently tied to `LocalStore`, it could probably even go in `libnixutil`. Functions not attached to `LocalStore` should not be declared in `local-store.hh`. I am moving it to facilitate experimenting for #9344. If canonicalisation should be done client-side in client-side builds, there wouldn't be a `LocalStore` at all so having to include that header to get this freestanding function is cumbersome and wrong. Perhaps canonicalisation should still be done server-side for security reasons --- I don't mean to make that judgement call now --- but even if so, this freestanding function still isn't connected to `LocalStore` so while less urgent it is still better to move out of this header.
46 lines
1.1 KiB
C++
46 lines
1.1 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include <sys/stat.h>
|
|
#include <sys/time.h>
|
|
|
|
#include "types.hh"
|
|
#include "error.hh"
|
|
|
|
namespace nix {
|
|
|
|
typedef std::pair<dev_t, ino_t> Inode;
|
|
typedef std::set<Inode> InodesSeen;
|
|
|
|
|
|
/**
|
|
* "Fix", or canonicalise, the meta-data of the files in a store path
|
|
* after it has been built. In particular:
|
|
*
|
|
* - the last modification date on each file is set to 1 (i.e.,
|
|
* 00:00:01 1/1/1970 UTC)
|
|
*
|
|
* - the permissions are set of 444 or 555 (i.e., read-only with or
|
|
* without execute permission; setuid bits etc. are cleared)
|
|
*
|
|
* - the owner and group are set to the Nix user and group, if we're
|
|
* running as root.
|
|
*
|
|
* If uidRange is not empty, this function will throw an error if it
|
|
* encounters files owned by a user outside of the closed interval
|
|
* [uidRange->first, uidRange->second].
|
|
*/
|
|
void canonicalisePathMetaData(
|
|
const Path & path,
|
|
std::optional<std::pair<uid_t, uid_t>> uidRange,
|
|
InodesSeen & inodesSeen);
|
|
void canonicalisePathMetaData(
|
|
const Path & path,
|
|
std::optional<std::pair<uid_t, uid_t>> uidRange);
|
|
|
|
void canonicaliseTimestampAndPermissions(const Path & path);
|
|
|
|
MakeError(PathInUse, Error);
|
|
|
|
}
|