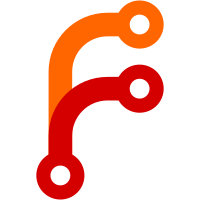
This adds a command 'nix make-content-addressable' that rewrites the specified store paths into content-addressable paths. The advantage of such paths is that 1) they can be imported without signatures; 2) they can enable deduplication in cases where derivation changes do not cause output changes (apart from store path hashes). For example, $ nix make-content-addressable -r nixpkgs.cowsay rewrote '/nix/store/g1g31ah55xdia1jdqabv1imf6mcw0nb1-glibc-2.25-49' to '/nix/store/48jfj7bg78a8n4f2nhg269rgw1936vj4-glibc-2.25-49' ... rewrote '/nix/store/qbi6rzpk0bxjw8lw6azn2mc7ynnn455q-cowsay-3.03+dfsg1-16' to '/nix/store/iq6g2x4q62xp7y7493bibx0qn5w7xz67-cowsay-3.03+dfsg1-16' We can then copy the resulting closure to another store without signatures: $ nix copy --trusted-public-keys '' ---to ~/my-nix /nix/store/iq6g2x4q62xp7y7493bibx0qn5w7xz67-cowsay-3.03+dfsg1-16 In order to support self-references in content-addressable paths, these paths are hashed "modulo" self-references, meaning that self-references are zeroed out during hashing. Somewhat annoyingly, this means that the NAR hash stored in the Nix database is no longer necessarily equal to the output of "nix hash-path"; for content-addressable paths, you need to pass the --modulo flag: $ nix path-info --json /nix/store/iq6g2x4q62xp7y7493bibx0qn5w7xz67-cowsay-3.03+dfsg1-16 | jq -r .[].narHash sha256:0ri611gdilz2c9rsibqhsipbfs9vwcqvs811a52i2bnkhv7w9mgw $ nix hash-path --type sha256 --base32 /nix/store/iq6g2x4q62xp7y7493bibx0qn5w7xz67-cowsay-3.03+dfsg1-16 1ggznh07khq0hz6id09pqws3a8q9pn03ya3c03nwck1kwq8rclzs $ nix hash-path --type sha256 --base32 /nix/store/iq6g2x4q62xp7y7493bibx0qn5w7xz67-cowsay-3.03+dfsg1-16 --modulo iq6g2x4q62xp7y7493bibx0qn5w7xz67 0ri611gdilz2c9rsibqhsipbfs9vwcqvs811a52i2bnkhv7w9mgw
39 lines
763 B
C++
39 lines
763 B
C++
#pragma once
|
|
|
|
#include "types.hh"
|
|
#include "hash.hh"
|
|
|
|
namespace nix {
|
|
|
|
PathSet scanForReferences(const Path & path, const PathSet & refs,
|
|
HashResult & hash);
|
|
|
|
struct RewritingSink : Sink
|
|
{
|
|
std::string from, to, prev;
|
|
Sink & nextSink;
|
|
uint64_t pos = 0;
|
|
|
|
std::vector<uint64_t> matches;
|
|
|
|
RewritingSink(const std::string & from, const std::string & to, Sink & nextSink);
|
|
|
|
void operator () (const unsigned char * data, size_t len) override;
|
|
|
|
void flush();
|
|
};
|
|
|
|
struct HashModuloSink : AbstractHashSink
|
|
{
|
|
HashSink hashSink;
|
|
RewritingSink rewritingSink;
|
|
|
|
HashModuloSink(HashType ht, const std::string & modulus);
|
|
|
|
void operator () (const unsigned char * data, size_t len) override;
|
|
|
|
HashResult finish() override;
|
|
};
|
|
|
|
}
|