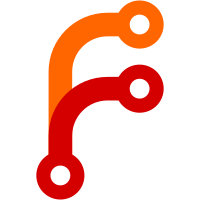
This does not yet resolve the coupling between packages and derivations, but it makes the code more consistent with the terminology, and it accentuates places where the coupling is obvious, such as auto drvPath = packageInfo.queryDrvPath(); if (!drvPath) throw Error("'%s' is not a derivation", what()); ... which isn't wrong, and in my opinion, doesn't even look wrong, because it just reflects the current logic. However, I do like that we can now start to see in the code that this coupling is perhaps a bit arbitrary. After this rename, we can bring the DerivingPath concept into type and start to lift this limitation.
104 lines
2.8 KiB
C++
104 lines
2.8 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include "eval.hh"
|
|
#include "path.hh"
|
|
|
|
#include <string>
|
|
#include <map>
|
|
|
|
|
|
namespace nix {
|
|
|
|
|
|
struct PackageInfo
|
|
{
|
|
public:
|
|
typedef std::map<std::string, std::optional<StorePath>> Outputs;
|
|
|
|
private:
|
|
EvalState * state;
|
|
|
|
mutable std::string name;
|
|
mutable std::string system;
|
|
mutable std::optional<std::optional<StorePath>> drvPath;
|
|
mutable std::optional<StorePath> outPath;
|
|
mutable std::string outputName;
|
|
Outputs outputs;
|
|
|
|
/**
|
|
* Set if we get an AssertionError
|
|
*/
|
|
bool failed = false;
|
|
|
|
Bindings * attrs = nullptr, * meta = nullptr;
|
|
|
|
Bindings * getMeta();
|
|
|
|
bool checkMeta(Value & v);
|
|
|
|
public:
|
|
/**
|
|
* path towards the derivation
|
|
*/
|
|
std::string attrPath;
|
|
|
|
PackageInfo(EvalState & state) : state(&state) { };
|
|
PackageInfo(EvalState & state, std::string attrPath, Bindings * attrs);
|
|
PackageInfo(EvalState & state, ref<Store> store, const std::string & drvPathWithOutputs);
|
|
|
|
std::string queryName() const;
|
|
std::string querySystem() const;
|
|
std::optional<StorePath> queryDrvPath() const;
|
|
StorePath requireDrvPath() const;
|
|
StorePath queryOutPath() const;
|
|
std::string queryOutputName() const;
|
|
/**
|
|
* Return the unordered map of output names to (optional) output paths.
|
|
* The "outputs to install" are determined by `meta.outputsToInstall`.
|
|
*/
|
|
Outputs queryOutputs(bool withPaths = true, bool onlyOutputsToInstall = false);
|
|
|
|
StringSet queryMetaNames();
|
|
Value * queryMeta(const std::string & name);
|
|
std::string queryMetaString(const std::string & name);
|
|
NixInt queryMetaInt(const std::string & name, NixInt def);
|
|
NixFloat queryMetaFloat(const std::string & name, NixFloat def);
|
|
bool queryMetaBool(const std::string & name, bool def);
|
|
void setMeta(const std::string & name, Value * v);
|
|
|
|
/*
|
|
MetaInfo queryMetaInfo(EvalState & state) const;
|
|
MetaValue queryMetaInfo(EvalState & state, const string & name) const;
|
|
*/
|
|
|
|
void setName(const std::string & s) { name = s; }
|
|
void setDrvPath(StorePath path) { drvPath = {{std::move(path)}}; }
|
|
void setOutPath(StorePath path) { outPath = {{std::move(path)}}; }
|
|
|
|
void setFailed() { failed = true; };
|
|
bool hasFailed() { return failed; };
|
|
};
|
|
|
|
|
|
#if HAVE_BOEHMGC
|
|
typedef std::list<PackageInfo, traceable_allocator<PackageInfo>> PackageInfos;
|
|
#else
|
|
typedef std::list<PackageInfo> PackageInfos;
|
|
#endif
|
|
|
|
|
|
/**
|
|
* If value `v` denotes a derivation, return a PackageInfo object
|
|
* describing it. Otherwise return nothing.
|
|
*/
|
|
std::optional<PackageInfo> getDerivation(EvalState & state,
|
|
Value & v, bool ignoreAssertionFailures);
|
|
|
|
void getDerivations(EvalState & state, Value & v, const std::string & pathPrefix,
|
|
Bindings & autoArgs, PackageInfos & drvs,
|
|
bool ignoreAssertionFailures);
|
|
|
|
|
|
}
|