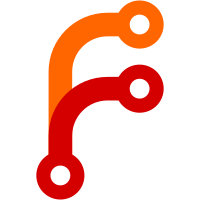
We want to be able to write down `foo.drv^bar.drv^baz`: `foo.drv^bar.drv` is the dynamic derivation (since it is itself a derivation output, `bar.drv` from `foo.drv`). To that end, we create `Single{Derivation,BuiltPath}` types, that are very similar except instead of having multiple outputs (in a set or map), they have a single one. This is for everything to the left of the rightmost `^`. `NixStringContextElem` has an analogous change, and now can reuse `SingleDerivedPath` at the top level. In fact, if we ever get rid of `DrvDeep`, `NixStringContextElem` could be replaced with `SingleDerivedPath` entirely! Important note: some JSON formats have changed. We already can *produce* dynamic derivations, but we can't refer to them directly. Today, we can merely express building or example at the top imperatively over time by building `foo.drv^bar.drv`, and then with a second nix invocation doing `<result-from-first>^baz`, but this is not declarative. The ethos of Nix of being able to write down the full plan everything you want to do, and then execute than plan with a single command, and for that we need the new inductive form of these types. Co-authored-by: Robert Hensing <roberth@users.noreply.github.com> Co-authored-by: Valentin Gagarin <valentin.gagarin@tweag.io>
69 lines
1.9 KiB
C++
69 lines
1.9 KiB
C++
#include "command.hh"
|
|
#include "common-args.hh"
|
|
#include "shared.hh"
|
|
#include "store-api.hh"
|
|
#include "log-store.hh"
|
|
#include "progress-bar.hh"
|
|
|
|
using namespace nix;
|
|
|
|
struct CmdLog : InstallableCommand
|
|
{
|
|
std::string description() override
|
|
{
|
|
return "show the build log of the specified packages or paths, if available";
|
|
}
|
|
|
|
std::string doc() override
|
|
{
|
|
return
|
|
#include "log.md"
|
|
;
|
|
}
|
|
|
|
Category category() override { return catSecondary; }
|
|
|
|
void run(ref<Store> store, ref<Installable> installable) override
|
|
{
|
|
settings.readOnlyMode = true;
|
|
|
|
auto subs = getDefaultSubstituters();
|
|
|
|
subs.push_front(store);
|
|
|
|
auto b = installable->toDerivedPath();
|
|
|
|
// For compat with CLI today, TODO revisit
|
|
auto oneUp = std::visit(overloaded {
|
|
[&](const DerivedPath::Opaque & bo) {
|
|
return make_ref<SingleDerivedPath>(bo);
|
|
},
|
|
[&](const DerivedPath::Built & bfd) {
|
|
return bfd.drvPath;
|
|
},
|
|
}, b.path.raw());
|
|
auto path = resolveDerivedPath(*store, *oneUp);
|
|
|
|
RunPager pager;
|
|
for (auto & sub : subs) {
|
|
auto * logSubP = dynamic_cast<LogStore *>(&*sub);
|
|
if (!logSubP) {
|
|
printInfo("Skipped '%s' which does not support retrieving build logs", sub->getUri());
|
|
continue;
|
|
}
|
|
auto & logSub = *logSubP;
|
|
|
|
auto log = logSub.getBuildLog(path);
|
|
if (!log) continue;
|
|
stopProgressBar();
|
|
printInfo("got build log for '%s' from '%s'", installable->what(), logSub.getUri());
|
|
writeFull(STDOUT_FILENO, *log);
|
|
return;
|
|
}
|
|
|
|
throw Error("build log of '%s' is not available", installable->what());
|
|
}
|
|
};
|
|
|
|
static auto rCmdLog = registerCommand<CmdLog>("log");
|