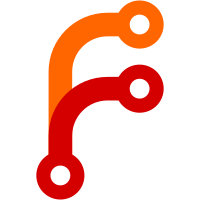
Ideally we would like to hide all other service's credentials for all services. That would imply for us to enable mount namespacing for all services, which is something we cannot do, both due to compatibility with the status quo ante, and because a number of services legitimately should be able to install mounts in the host hierarchy. Hence we do the second best thing, we hide the credentials automatically for all services that opt into mount namespacing otherwise. This is quite different from other mount sandboxing options: usually you have to explicitly opt into each. However, given that the credentials logic is a brand new concept we invented right here and now, and particularly security sensitive it's OK to reverse this, and by default hide credentials whenever we can (i.e. whenever mount namespacing is otherwise opt-ed in to). Long story short: if you want to hide other service's credentials, the most basic options is to just turn on PrivateMounts= and there you go, they should all be gone.
107 lines
3.4 KiB
C
107 lines
3.4 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
|
|
#include <errno.h>
|
|
#include <stdlib.h>
|
|
#include <unistd.h>
|
|
|
|
#include "log.h"
|
|
#include "namespace.h"
|
|
#include "tests.h"
|
|
|
|
int main(int argc, char *argv[]) {
|
|
const char * const writable[] = {
|
|
"/home",
|
|
"-/home/lennart/projects/foobar", /* this should be masked automatically */
|
|
NULL
|
|
};
|
|
|
|
const char * const readonly[] = {
|
|
/* "/", */
|
|
/* "/usr", */
|
|
"/boot",
|
|
"/lib",
|
|
"/usr/lib",
|
|
"-/lib64",
|
|
"-/usr/lib64",
|
|
NULL
|
|
};
|
|
|
|
const char *inaccessible[] = {
|
|
"/home/lennart/projects",
|
|
NULL
|
|
};
|
|
|
|
static const NamespaceInfo ns_info = {
|
|
.private_dev = true,
|
|
.protect_control_groups = true,
|
|
.protect_kernel_tunables = true,
|
|
.protect_kernel_modules = true,
|
|
.protect_proc = PROTECT_PROC_NOACCESS,
|
|
.proc_subset = PROC_SUBSET_PID,
|
|
};
|
|
|
|
char *root_directory;
|
|
char *projects_directory;
|
|
int r;
|
|
char tmp_dir[] = "/tmp/systemd-private-XXXXXX",
|
|
var_tmp_dir[] = "/var/tmp/systemd-private-XXXXXX";
|
|
|
|
test_setup_logging(LOG_DEBUG);
|
|
|
|
assert_se(mkdtemp(tmp_dir));
|
|
assert_se(mkdtemp(var_tmp_dir));
|
|
|
|
root_directory = getenv("TEST_NS_CHROOT");
|
|
projects_directory = getenv("TEST_NS_PROJECTS");
|
|
|
|
if (projects_directory)
|
|
inaccessible[0] = projects_directory;
|
|
|
|
log_info("Inaccessible directory: '%s'", inaccessible[0]);
|
|
if (root_directory)
|
|
log_info("Chroot: '%s'", root_directory);
|
|
else
|
|
log_info("Not chrooted");
|
|
|
|
r = setup_namespace(root_directory,
|
|
NULL,
|
|
NULL,
|
|
&ns_info,
|
|
(char **) writable,
|
|
(char **) readonly,
|
|
(char **) inaccessible,
|
|
NULL,
|
|
&(BindMount) { .source = (char*) "/usr/bin", .destination = (char*) "/etc/systemd", .read_only = true }, 1,
|
|
&(TemporaryFileSystem) { .path = (char*) "/var", .options = (char*) "ro" }, 1,
|
|
NULL,
|
|
0,
|
|
tmp_dir,
|
|
var_tmp_dir,
|
|
NULL,
|
|
NULL,
|
|
0,
|
|
NULL,
|
|
0,
|
|
NULL,
|
|
NULL,
|
|
0,
|
|
NULL,
|
|
NULL,
|
|
0,
|
|
NULL);
|
|
if (r < 0) {
|
|
log_error_errno(r, "Failed to set up namespace: %m");
|
|
|
|
log_info("Usage:\n"
|
|
" sudo TEST_NS_PROJECTS=/home/lennart/projects ./test-ns\n"
|
|
" sudo TEST_NS_CHROOT=/home/alban/debian-tree TEST_NS_PROJECTS=/home/alban/debian-tree/home/alban/Documents ./test-ns");
|
|
|
|
return 1;
|
|
}
|
|
|
|
execl("/bin/sh", "/bin/sh", NULL);
|
|
log_error_errno(errno, "execl(): %m");
|
|
|
|
return 1;
|
|
}
|