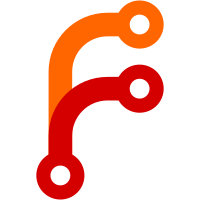
Also called "ANSI-C Quoting" in info:(bash) ANSI-C Quoting. The escaping rules are a POSIX proposal, and are described in http://austingroupbugs.net/view.php?id=249. There's a lot of back-and-forth on the details of escaping of control characters, but we'll be only using a small subset of the syntax that is common to all proposals and is widely supported. Unfortunately dash and fish and maybe some other shells do not support it (see the man page patch for a list). This allows environment variables to be safely exported using show-environment and imported into the shell. Shells which do not support this syntax will have to do something like export $(systemctl show-environment|grep -v '=\$') or whatever is appropriate in their case. I think csh and fish do not support the A=B syntax anyway, so the change is moot for them. Fixes #5536. v2: - also escape newlines (which currently disallowed in shell values, so this doesn't really matter), and tabs (as $'\t'), and ! (as $'!'). This way quoted output can be included directly in both interactive and noninteractive bash.
72 lines
2.4 KiB
C
72 lines
2.4 KiB
C
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2010 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
#include <inttypes.h>
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
#include <sys/types.h>
|
|
#include <uchar.h>
|
|
|
|
#include "string-util.h"
|
|
#include "missing.h"
|
|
|
|
/* What characters are special in the shell? */
|
|
/* must be escaped outside and inside double-quotes */
|
|
#define SHELL_NEED_ESCAPE "\"\\`$"
|
|
|
|
/* Those that can be escaped or double-quoted.
|
|
*
|
|
* Stricly speaking, ! does not need to be escaped, except in interactive
|
|
* mode, but let's be extra nice to the user and quote ! in case this
|
|
* output is ever used in interactive mode. */
|
|
#define SHELL_NEED_QUOTES SHELL_NEED_ESCAPE GLOB_CHARS "'()<>|&;!"
|
|
|
|
/* Note that we assume control characters would need to be escaped too in
|
|
* addition to the "special" characters listed here, if they appear in the
|
|
* string. Current users disallow control characters. Also '"' shall not
|
|
* be escaped.
|
|
*/
|
|
#define SHELL_NEED_ESCAPE_POSIX "\\\'"
|
|
|
|
typedef enum UnescapeFlags {
|
|
UNESCAPE_RELAX = 1,
|
|
} UnescapeFlags;
|
|
|
|
typedef enum EscapeStyle {
|
|
ESCAPE_BACKSLASH = 1,
|
|
ESCAPE_POSIX = 2,
|
|
} EscapeStyle;
|
|
|
|
char *cescape(const char *s);
|
|
char *cescape_length(const char *s, size_t n);
|
|
size_t cescape_char(char c, char *buf);
|
|
|
|
int cunescape(const char *s, UnescapeFlags flags, char **ret);
|
|
int cunescape_length(const char *s, size_t length, UnescapeFlags flags, char **ret);
|
|
int cunescape_length_with_prefix(const char *s, size_t length, const char *prefix, UnescapeFlags flags, char **ret);
|
|
int cunescape_one(const char *p, size_t length, char32_t *ret, bool *eight_bit);
|
|
|
|
char *xescape(const char *s, const char *bad);
|
|
char *octescape(const char *s, size_t len);
|
|
|
|
char *shell_escape(const char *s, const char *bad);
|
|
char* shell_maybe_quote(const char *s, EscapeStyle style);
|