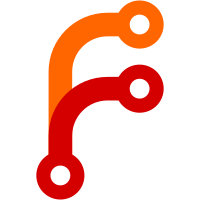
This patch improves parsing and generation of timestamps and calendar specifications in two ways: - The week day is now always printed in the abbreviated English form, instead of the locale's setting. This makes sure we can always parse the week day again, even if the locale is changed. Given that we don't follow locale settings for printing timestamps in any other way either (for example, we always use 24h syntax in order to make uniform parsing possible), it only makes sense to also stick to a generic, non-localized form for the timestamp, too. - When parsing a timestamp, the local timezone (in its DST or non-DST name) may be specified, in addition to "UTC". Other timezones are still not supported however (not because we wouldn't want to, but mostly because libc offers no nice API for that). In itself this brings no new features, however it ensures that any locally formatted timestamp's timezone is also parsable again. These two changes ensure that the output of format_timestamp() may always be passed to parse_timestamp() and results in the original input. The related flavours for usec/UTC also work accordingly. Calendar specifications are extended in a similar way. The man page is updated accordingly, in particular this removes the claim that timestamps systemd prints wouldn't be parsable by systemd. They are now. The man page previously showed invalid timestamps as examples. This has been removed, as the man page shouldn't be a unit test, where such negative examples would be useful. The man page also no longer mentions the names of internal functions, such as format_timestamp_us() or UNIX error codes such as EINVAL.
183 lines
7.4 KiB
C
183 lines
7.4 KiB
C
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2012 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
#include <string.h>
|
|
|
|
#include "alloc-util.h"
|
|
#include "calendarspec.h"
|
|
#include "string-util.h"
|
|
#include "util.h"
|
|
|
|
static void test_one(const char *input, const char *output) {
|
|
CalendarSpec *c;
|
|
_cleanup_free_ char *p = NULL, *q = NULL;
|
|
usec_t u;
|
|
char buf[FORMAT_TIMESTAMP_MAX];
|
|
int r;
|
|
|
|
assert_se(calendar_spec_from_string(input, &c) >= 0);
|
|
|
|
assert_se(calendar_spec_to_string(c, &p) >= 0);
|
|
printf("\"%s\" → \"%s\"\n", input, p);
|
|
|
|
assert_se(streq(p, output));
|
|
|
|
u = now(CLOCK_REALTIME);
|
|
r = calendar_spec_next_usec(c, u, &u);
|
|
printf("Next: %s\n", r < 0 ? strerror(-r) : format_timestamp(buf, sizeof(buf), u));
|
|
calendar_spec_free(c);
|
|
|
|
assert_se(calendar_spec_from_string(p, &c) >= 0);
|
|
assert_se(calendar_spec_to_string(c, &q) >= 0);
|
|
calendar_spec_free(c);
|
|
|
|
assert_se(streq(q, p));
|
|
}
|
|
|
|
static void test_next(const char *input, const char *new_tz, usec_t after, usec_t expect) {
|
|
CalendarSpec *c;
|
|
usec_t u;
|
|
char *old_tz;
|
|
char buf[FORMAT_TIMESTAMP_MAX];
|
|
int r;
|
|
|
|
old_tz = getenv("TZ");
|
|
if (old_tz)
|
|
old_tz = strdupa(old_tz);
|
|
|
|
if (new_tz)
|
|
assert_se(setenv("TZ", new_tz, 1) >= 0);
|
|
else
|
|
assert_se(unsetenv("TZ") >= 0);
|
|
tzset();
|
|
|
|
assert_se(calendar_spec_from_string(input, &c) >= 0);
|
|
|
|
printf("\"%s\"\n", input);
|
|
|
|
u = after;
|
|
r = calendar_spec_next_usec(c, after, &u);
|
|
printf("At: %s\n", r < 0 ? strerror(-r) : format_timestamp_us(buf, sizeof(buf), u));
|
|
if (expect != (usec_t)-1)
|
|
assert_se(r >= 0 && u == expect);
|
|
else
|
|
assert(r == -ENOENT);
|
|
|
|
calendar_spec_free(c);
|
|
|
|
if (old_tz)
|
|
assert_se(setenv("TZ", old_tz, 1) >= 0);
|
|
else
|
|
assert_se(unsetenv("TZ") >= 0);
|
|
tzset();
|
|
}
|
|
|
|
static void test_timestamp(void) {
|
|
char buf[FORMAT_TIMESTAMP_MAX];
|
|
_cleanup_free_ char *t = NULL;
|
|
CalendarSpec *c;
|
|
usec_t x, y;
|
|
|
|
/* Ensure that a timestamp is also a valid calendar specification. Convert forth and back */
|
|
|
|
x = now(CLOCK_REALTIME);
|
|
|
|
assert_se(format_timestamp_us(buf, sizeof(buf), x));
|
|
printf("%s\n", buf);
|
|
assert_se(calendar_spec_from_string(buf, &c) >= 0);
|
|
assert_se(calendar_spec_to_string(c, &t) >= 0);
|
|
calendar_spec_free(c);
|
|
printf("%s\n", t);
|
|
|
|
assert_se(parse_timestamp(t, &y) >= 0);
|
|
assert_se(y == x);
|
|
}
|
|
|
|
int main(int argc, char* argv[]) {
|
|
CalendarSpec *c;
|
|
|
|
test_one("Sat,Thu,Mon-Wed,Sat-Sun", "Mon..Thu,Sat,Sun *-*-* 00:00:00");
|
|
test_one("Sat,Thu,Mon..Wed,Sat..Sun", "Mon..Thu,Sat,Sun *-*-* 00:00:00");
|
|
test_one("Mon,Sun 12-*-* 2,1:23", "Mon,Sun 2012-*-* 01,02:23:00");
|
|
test_one("Wed *-1", "Wed *-*-01 00:00:00");
|
|
test_one("Wed-Wed,Wed *-1", "Wed *-*-01 00:00:00");
|
|
test_one("Wed..Wed,Wed *-1", "Wed *-*-01 00:00:00");
|
|
test_one("Wed, 17:48", "Wed *-*-* 17:48:00");
|
|
test_one("Wed-Sat,Tue 12-10-15 1:2:3", "Tue..Sat 2012-10-15 01:02:03");
|
|
test_one("Wed..Sat,Tue 12-10-15 1:2:3", "Tue..Sat 2012-10-15 01:02:03");
|
|
test_one("*-*-7 0:0:0", "*-*-07 00:00:00");
|
|
test_one("10-15", "*-10-15 00:00:00");
|
|
test_one("monday *-12-* 17:00", "Mon *-12-* 17:00:00");
|
|
test_one("Mon,Fri *-*-3,1,2 *:30:45", "Mon,Fri *-*-01,02,03 *:30:45");
|
|
test_one("12,14,13,12:20,10,30", "*-*-* 12,13,14:10,20,30:00");
|
|
test_one("mon,fri *-1/2-1,3 *:30:45", "Mon,Fri *-01/2-01,03 *:30:45");
|
|
test_one("03-05 08:05:40", "*-03-05 08:05:40");
|
|
test_one("08:05:40", "*-*-* 08:05:40");
|
|
test_one("05:40", "*-*-* 05:40:00");
|
|
test_one("Sat,Sun 12-05 08:05:40", "Sat,Sun *-12-05 08:05:40");
|
|
test_one("Sat,Sun 08:05:40", "Sat,Sun *-*-* 08:05:40");
|
|
test_one("2003-03-05 05:40", "2003-03-05 05:40:00");
|
|
test_one("2003-03-05", "2003-03-05 00:00:00");
|
|
test_one("03-05", "*-03-05 00:00:00");
|
|
test_one("hourly", "*-*-* *:00:00");
|
|
test_one("daily", "*-*-* 00:00:00");
|
|
test_one("monthly", "*-*-01 00:00:00");
|
|
test_one("weekly", "Mon *-*-* 00:00:00");
|
|
test_one("minutely", "*-*-* *:*:00");
|
|
test_one("quarterly", "*-01,04,07,10-01 00:00:00");
|
|
test_one("semi-annually", "*-01,07-01 00:00:00");
|
|
test_one("annually", "*-01-01 00:00:00");
|
|
test_one("*:2/3", "*-*-* *:02/3:00");
|
|
test_one("2015-10-25 01:00:00 uTc", "2015-10-25 01:00:00 UTC");
|
|
test_one("2016-03-27 03:17:00.4200005", "2016-03-27 03:17:00.420001");
|
|
test_one("2016-03-27 03:17:00/0.42", "2016-03-27 03:17:00/0.420000");
|
|
test_one("2016-03-27 03:17:00/0.42", "2016-03-27 03:17:00/0.420000");
|
|
test_one("9..11,13:00,30", "*-*-* 09,10,11,13:00,30:00");
|
|
test_one("1..3-1..3 1..3:1..3", "*-01,02,03-01,02,03 01,02,03:01,02,03:00");
|
|
test_one("00:00:1.125..2.125", "*-*-* 00:00:01.125000,02.125000");
|
|
test_one("00:00:1.0..3.8", "*-*-* 00:00:01,02,03");
|
|
|
|
test_next("2016-03-27 03:17:00", "", 12345, 1459048620000000);
|
|
test_next("2016-03-27 03:17:00", "CET", 12345, 1459041420000000);
|
|
test_next("2016-03-27 03:17:00", "EET", 12345, -1);
|
|
test_next("2016-03-27 03:17:00 UTC", NULL, 12345, 1459048620000000);
|
|
test_next("2016-03-27 03:17:00 UTC", "", 12345, 1459048620000000);
|
|
test_next("2016-03-27 03:17:00 UTC", "CET", 12345, 1459048620000000);
|
|
test_next("2016-03-27 03:17:00 UTC", "EET", 12345, 1459048620000000);
|
|
test_next("2016-03-27 03:17:00.420000001 UTC", "EET", 12345, 1459048620420000);
|
|
test_next("2016-03-27 03:17:00.4200005 UTC", "EET", 12345, 1459048620420001);
|
|
test_next("2015-11-13 09:11:23.42", "EET", 12345, 1447398683420000);
|
|
test_next("2015-11-13 09:11:23.42/1.77", "EET", 1447398683420000, 1447398685190000);
|
|
test_next("2015-11-13 09:11:23.42/1.77", "EET", 1447398683419999, 1447398683420000);
|
|
test_next("Sun 16:00:00", "CET", 1456041600123456, 1456066800000000);
|
|
|
|
assert_se(calendar_spec_from_string("test", &c) < 0);
|
|
assert_se(calendar_spec_from_string("", &c) < 0);
|
|
assert_se(calendar_spec_from_string("7", &c) < 0);
|
|
assert_se(calendar_spec_from_string("121212:1:2", &c) < 0);
|
|
assert_se(calendar_spec_from_string("2000-03-05.23 00:00:00", &c) < 0);
|
|
assert_se(calendar_spec_from_string("2000-03-05 00:00.1:00", &c) < 0);
|
|
assert_se(calendar_spec_from_string("00:00:00/0.00000001", &c) < 0);
|
|
assert_se(calendar_spec_from_string("00:00:00.0..00.9", &c) < 0);
|
|
|
|
test_timestamp();
|
|
|
|
return 0;
|
|
}
|