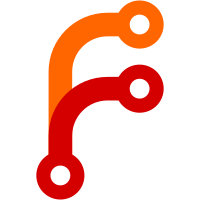
This doesn't have much effect on the final build, because we link libbasic.a into libsystemd-shared.so, so in the end, all the object built from basic/ end up in libsystemd-shared. And when the static library is linked into binaries, any objects that are included in it but are not used are trimmed. Hence, the size of output artifacts doesn't change: $ du -sb /var/tmp/inst* 54181861 /var/tmp/inst1 (old) 54207441 /var/tmp/inst1s (old split-usr) 54182477 /var/tmp/inst2 (new) 54208041 /var/tmp/inst2s (new split-usr) (The negligible change in size is because libsystemd-shared.so is bigger by a few hundred bytes. I guess it's because symbols are named differently or something like that.) The effect is on the build process, in particular partial builds. This change effectively moves the requirements on some build steps toward the leaves of the dependency tree. Two effects: - when building items that do not depend on libsystemd-shared, we build less stuff for libbasic.a (which wouldn't be used anyway, so it's a net win). - when building items that do depend on libshared, we reduce libbasic.a as a synchronization point, possibly allowing better parallelism. Method: 1. copy list of .h files from src/basic/meson.build to /tmp/basic 2. $ for i in $(grep '.h$' /tmp/basic); do echo $i; git --no-pager grep "include \"$i\"" src/basic/ 'src/lib*' 'src/nss-*' 'src/journal/sd-journal.c' |grep -v "${i%.h}.c";echo ;done | less
64 lines
2.5 KiB
C
64 lines
2.5 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
|
|
#pragma once
|
|
|
|
#include "json.h"
|
|
|
|
/* This header should include all prototypes only the JSON parser itself and
|
|
* its tests need access to. Normal code consuming the JSON parser should not
|
|
* interface with this. */
|
|
|
|
typedef union JsonValue {
|
|
/* Encodes a simple value. On x86-64 this structure is 16 bytes wide (as long double is 128bit). */
|
|
bool boolean;
|
|
long double real;
|
|
intmax_t integer;
|
|
uintmax_t unsig;
|
|
} JsonValue;
|
|
|
|
/* Let's protect us against accidental structure size changes on our most relevant arch */
|
|
#ifdef __x86_64__
|
|
assert_cc(sizeof(JsonValue) == 16U);
|
|
#endif
|
|
|
|
#define JSON_VALUE_NULL ((JsonValue) {})
|
|
|
|
/* We use fake JsonVariant objects for some special values, in order to avoid memory allocations for them. Note that
|
|
* effectively this means that there are multiple ways to encode the same objects: via these magic values or as
|
|
* properly allocated JsonVariant. We convert between both on-the-fly as necessary. */
|
|
#define JSON_VARIANT_MAGIC_TRUE ((JsonVariant*) 1)
|
|
#define JSON_VARIANT_MAGIC_FALSE ((JsonVariant*) 2)
|
|
#define JSON_VARIANT_MAGIC_NULL ((JsonVariant*) 3)
|
|
#define JSON_VARIANT_MAGIC_ZERO_INTEGER ((JsonVariant*) 4)
|
|
#define JSON_VARIANT_MAGIC_ZERO_UNSIGNED ((JsonVariant*) 5)
|
|
#define JSON_VARIANT_MAGIC_ZERO_REAL ((JsonVariant*) 6)
|
|
#define JSON_VARIANT_MAGIC_EMPTY_STRING ((JsonVariant*) 7)
|
|
#define JSON_VARIANT_MAGIC_EMPTY_ARRAY ((JsonVariant*) 8)
|
|
#define JSON_VARIANT_MAGIC_EMPTY_OBJECT ((JsonVariant*) 9)
|
|
#define _JSON_VARIANT_MAGIC_MAX ((JsonVariant*) 10)
|
|
|
|
/* This is only safe as long as we don't define more than 4K magic pointers, i.e. the page size of the simplest
|
|
* architectures we support. That's because we rely on the fact that malloc() will never allocate from the first memory
|
|
* page, as it is a faulting page for catching NULL pointer dereferences. */
|
|
assert_cc((uintptr_t) _JSON_VARIANT_MAGIC_MAX < 4096U);
|
|
|
|
enum { /* JSON tokens */
|
|
JSON_TOKEN_END,
|
|
JSON_TOKEN_COLON,
|
|
JSON_TOKEN_COMMA,
|
|
JSON_TOKEN_OBJECT_OPEN,
|
|
JSON_TOKEN_OBJECT_CLOSE,
|
|
JSON_TOKEN_ARRAY_OPEN,
|
|
JSON_TOKEN_ARRAY_CLOSE,
|
|
JSON_TOKEN_STRING,
|
|
JSON_TOKEN_REAL,
|
|
JSON_TOKEN_INTEGER,
|
|
JSON_TOKEN_UNSIGNED,
|
|
JSON_TOKEN_BOOLEAN,
|
|
JSON_TOKEN_NULL,
|
|
_JSON_TOKEN_MAX,
|
|
_JSON_TOKEN_INVALID = -1,
|
|
};
|
|
|
|
int json_tokenize(const char **p, char **ret_string, JsonValue *ret_value, unsigned *ret_line, unsigned *ret_column, void **state, unsigned *line, unsigned *column);
|