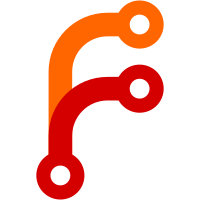
This drops a good number of type-specific _cleanup_ macros, and patches all users to just use the generic ones. In most recent code we abstained from defining type-specific macros, and this basically removes all those added already, with the exception of the really low-level ones. Having explicit macros for this is not too useful, as the expression without the extra macro is generally just 2ch wider. We should generally emphesize generic code, unless there are really good reasons for specific code, hence let's follow this in this case too. Note that _cleanup_free_ and similar really low-level, libc'ish, Linux API'ish macros continue to be defined, only the really high-level OO ones are dropped. From now on this should really be the rule: for really low-level stuff, such as memory allocation, fd handling and so one, go ahead and define explicit per-type macros, but for high-level, specific program code, just use the generic _cleanup_() macro directly, in order to keep things simple and as readable as possible for the uninitiated. Note that before this patch some of the APIs (notable libudev ones) were already used with the high-level macros at some places and with the generic _cleanup_ macro at others. With this patch we hence unify on the latter.
76 lines
4.7 KiB
C
76 lines
4.7 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2013 Tom Gundersen <teg@jklm.no>
|
|
***/
|
|
|
|
typedef struct Route Route;
|
|
typedef struct NetworkConfigSection NetworkConfigSection;
|
|
|
|
#include "networkd-network.h"
|
|
|
|
struct Route {
|
|
Network *network;
|
|
NetworkConfigSection *section;
|
|
|
|
Link *link;
|
|
|
|
int family;
|
|
int quickack;
|
|
|
|
unsigned char dst_prefixlen;
|
|
unsigned char src_prefixlen;
|
|
unsigned char scope;
|
|
unsigned char protocol; /* RTPROT_* */
|
|
unsigned char type; /* RTN_* */
|
|
unsigned char tos;
|
|
uint32_t priority; /* note that ip(8) calls this 'metric' */
|
|
uint32_t table;
|
|
uint32_t mtu;
|
|
uint32_t initcwnd;
|
|
uint32_t initrwnd;
|
|
unsigned char pref;
|
|
unsigned flags;
|
|
|
|
union in_addr_union gw;
|
|
union in_addr_union dst;
|
|
union in_addr_union src;
|
|
union in_addr_union prefsrc;
|
|
|
|
usec_t lifetime;
|
|
sd_event_source *expire;
|
|
|
|
LIST_FIELDS(Route, routes);
|
|
};
|
|
|
|
int route_new_static(Network *network, const char *filename, unsigned section_line, Route **ret);
|
|
int route_new(Route **ret);
|
|
void route_free(Route *route);
|
|
int route_configure(Route *route, Link *link, sd_netlink_message_handler_t callback);
|
|
int route_remove(Route *route, Link *link, sd_netlink_message_handler_t callback);
|
|
|
|
int route_get(Link *link, int family, const union in_addr_union *dst, unsigned char dst_prefixlen, unsigned char tos, uint32_t priority, uint32_t table, Route **ret);
|
|
int route_add(Link *link, int family, const union in_addr_union *dst, unsigned char dst_prefixlen, unsigned char tos, uint32_t priority, uint32_t table, Route **ret);
|
|
int route_add_foreign(Link *link, int family, const union in_addr_union *dst, unsigned char dst_prefixlen, unsigned char tos, uint32_t priority, uint32_t table, Route **ret);
|
|
void route_update(Route *route, const union in_addr_union *src, unsigned char src_prefixlen, const union in_addr_union *gw, const union in_addr_union *prefsrc, unsigned char scope, unsigned char protocol, unsigned char type);
|
|
|
|
int route_expire_handler(sd_event_source *s, uint64_t usec, void *userdata);
|
|
|
|
DEFINE_TRIVIAL_CLEANUP_FUNC(Route*, route_free);
|
|
|
|
int config_parse_gateway(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_preferred_src(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_destination(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_route_priority(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_route_scope(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_route_table(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_gateway_onlink(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_ipv6_route_preference(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_route_protocol(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_route_type(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_tcp_window(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|
|
int config_parse_quickack(const char *unit, const char *filename, unsigned line, const char *section, unsigned section_line, const char *lvalue, int ltype, const char *rvalue, void *data, void *userdata);
|