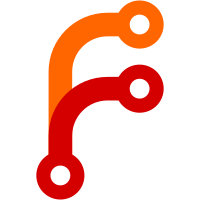
This adds IOVEC_INIT() and IOVEC_MAKE() for initializing iovec structures from a pointer and a size. On top of these IOVEC_INIT_STRING() and IOVEC_MAKE_STRING() are added which take a string and automatically determine the size of the string using strlen(). This patch removes the old IOVEC_SET_STRING() macro, given that IOVEC_MAKE_STRING() is now useful for similar purposes. Note that the old IOVEC_SET_STRING() invocations were two characters shorter than the new ones using IOVEC_MAKE_STRING(), but I think the new syntax is more readable and more generic as it simply resolves to a C99 literal structure initialization. Moreover, we can use very similar syntax now for initializing strings and pointer+size iovec entries. We canalso use the new macros to initialize function parameters on-the-fly or array definitions. And given that we shouldn't have so many ways to do the same stuff, let's just settle on the new macros. (This also converts some code to use _cleanup_ where dynamically allocated strings were using IOVEC_SET_STRING() before, to modernize things a bit)
93 lines
2.7 KiB
C
93 lines
2.7 KiB
C
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2010 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
#include <stdbool.h>
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
#include <sys/types.h>
|
|
#include <sys/uio.h>
|
|
|
|
#include "macro.h"
|
|
#include "time-util.h"
|
|
|
|
int flush_fd(int fd);
|
|
|
|
ssize_t loop_read(int fd, void *buf, size_t nbytes, bool do_poll);
|
|
int loop_read_exact(int fd, void *buf, size_t nbytes, bool do_poll);
|
|
int loop_write(int fd, const void *buf, size_t nbytes, bool do_poll);
|
|
|
|
int pipe_eof(int fd);
|
|
|
|
int fd_wait_for_event(int fd, int event, usec_t timeout);
|
|
|
|
ssize_t sparse_write(int fd, const void *p, size_t sz, size_t run_length);
|
|
|
|
static inline size_t IOVEC_TOTAL_SIZE(const struct iovec *i, unsigned n) {
|
|
unsigned j;
|
|
size_t r = 0;
|
|
|
|
for (j = 0; j < n; j++)
|
|
r += i[j].iov_len;
|
|
|
|
return r;
|
|
}
|
|
|
|
static inline size_t IOVEC_INCREMENT(struct iovec *i, unsigned n, size_t k) {
|
|
unsigned j;
|
|
|
|
for (j = 0; j < n; j++) {
|
|
size_t sub;
|
|
|
|
if (_unlikely_(k <= 0))
|
|
break;
|
|
|
|
sub = MIN(i[j].iov_len, k);
|
|
i[j].iov_len -= sub;
|
|
i[j].iov_base = (uint8_t*) i[j].iov_base + sub;
|
|
k -= sub;
|
|
}
|
|
|
|
return k;
|
|
}
|
|
|
|
static inline bool FILE_SIZE_VALID(uint64_t l) {
|
|
/* ftruncate() and friends take an unsigned file size, but actually cannot deal with file sizes larger than
|
|
* 2^63 since the kernel internally handles it as signed value. This call allows checking for this early. */
|
|
|
|
return (l >> 63) == 0;
|
|
}
|
|
|
|
static inline bool FILE_SIZE_VALID_OR_INFINITY(uint64_t l) {
|
|
|
|
/* Same as above, but allows one extra value: -1 as indication for infinity. */
|
|
|
|
if (l == (uint64_t) -1)
|
|
return true;
|
|
|
|
return FILE_SIZE_VALID(l);
|
|
|
|
}
|
|
|
|
#define IOVEC_INIT(base, len) { .iov_base = (base), .iov_len = (len) }
|
|
#define IOVEC_MAKE(base, len) (struct iovec) IOVEC_INIT(base, len)
|
|
#define IOVEC_INIT_STRING(string) IOVEC_INIT((char*) string, strlen(string))
|
|
#define IOVEC_MAKE_STRING(string) (struct iovec) IOVEC_INIT_STRING(string)
|