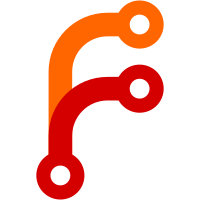
Given two bitmaps and the following code: Bitmap *a = bitmap_new(), *b = bitmap_new(); bitmap_set(a, 1); bitmap_clear(a); bitmap_set(a, 0); bitmap_set(b, 0); These two bitmaps should now have the same bits set and they should be equal but bitmap_equal() will return false in this case because while bitmap_clear() resets the number of elements in the array it does not clear the array and bitmap_set() expects the array to be cleared. GREEDY_REALLOC0 looks at the allocated size and not the actual size so it does not clear any memory. Fix this by freeing the allocated memory and resetting the whole Bitmap to an initial state in bitmap_clear(). This also adds test code for this issue.
124 lines
3.7 KiB
C
124 lines
3.7 KiB
C
/***
|
|
This file is part of systemd
|
|
|
|
Copyright 2015 Tom Gundersen
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
#include "bitmap.h"
|
|
|
|
int main(int argc, const char *argv[]) {
|
|
_cleanup_bitmap_free_ Bitmap *b = NULL, *b2 = NULL;
|
|
Iterator it;
|
|
unsigned n = (unsigned) -1, i = 0;
|
|
|
|
b = bitmap_new();
|
|
assert_se(b);
|
|
|
|
assert_se(bitmap_ensure_allocated(&b) == 0);
|
|
bitmap_free(b);
|
|
b = NULL;
|
|
assert_se(bitmap_ensure_allocated(&b) == 0);
|
|
|
|
assert_se(bitmap_isset(b, 0) == false);
|
|
assert_se(bitmap_isset(b, 1) == false);
|
|
assert_se(bitmap_isset(b, 256) == false);
|
|
assert_se(bitmap_isclear(b) == true);
|
|
|
|
assert_se(bitmap_set(b, 0) == 0);
|
|
assert_se(bitmap_isset(b, 0) == true);
|
|
assert_se(bitmap_isclear(b) == false);
|
|
bitmap_unset(b, 0);
|
|
assert_se(bitmap_isset(b, 0) == false);
|
|
assert_se(bitmap_isclear(b) == true);
|
|
|
|
assert_se(bitmap_set(b, 1) == 0);
|
|
assert_se(bitmap_isset(b, 1) == true);
|
|
assert_se(bitmap_isclear(b) == false);
|
|
bitmap_unset(b, 1);
|
|
assert_se(bitmap_isset(b, 1) == false);
|
|
assert_se(bitmap_isclear(b) == true);
|
|
|
|
assert_se(bitmap_set(b, 256) == 0);
|
|
assert_se(bitmap_isset(b, 256) == true);
|
|
assert_se(bitmap_isclear(b) == false);
|
|
bitmap_unset(b, 256);
|
|
assert_se(bitmap_isset(b, 256) == false);
|
|
assert_se(bitmap_isclear(b) == true);
|
|
|
|
assert_se(bitmap_set(b, 32) == 0);
|
|
bitmap_unset(b, 0);
|
|
assert_se(bitmap_isset(b, 32) == true);
|
|
bitmap_unset(b, 32);
|
|
|
|
BITMAP_FOREACH(n, NULL, it)
|
|
assert_not_reached("NULL bitmap");
|
|
|
|
assert_se(bitmap_set(b, 0) == 0);
|
|
assert_se(bitmap_set(b, 1) == 0);
|
|
assert_se(bitmap_set(b, 256) == 0);
|
|
|
|
BITMAP_FOREACH(n, b, it) {
|
|
assert_se(n == i);
|
|
if (i == 0)
|
|
i = 1;
|
|
else if (i == 1)
|
|
i = 256;
|
|
else if (i == 256)
|
|
i = (unsigned) -1;
|
|
}
|
|
|
|
assert_se(i == (unsigned) -1);
|
|
|
|
i = 0;
|
|
|
|
BITMAP_FOREACH(n, b, it) {
|
|
assert_se(n == i);
|
|
if (i == 0)
|
|
i = 1;
|
|
else if (i == 1)
|
|
i = 256;
|
|
else if (i == 256)
|
|
i = (unsigned) -1;
|
|
}
|
|
|
|
assert_se(i == (unsigned) -1);
|
|
|
|
bitmap_clear(b);
|
|
assert_se(bitmap_isclear(b) == true);
|
|
|
|
assert_se(bitmap_set(b, (unsigned) -1) == -ERANGE);
|
|
|
|
bitmap_free(b);
|
|
b = NULL;
|
|
assert_se(bitmap_ensure_allocated(&b) == 0);
|
|
assert_se(bitmap_ensure_allocated(&b2) == 0);
|
|
|
|
assert_se(bitmap_equal(b, b2));
|
|
assert_se(bitmap_set(b, 0) == 0);
|
|
bitmap_unset(b, 0);
|
|
assert_se(bitmap_equal(b, b2));
|
|
|
|
assert_se(bitmap_set(b, 1) == 0);
|
|
bitmap_clear(b);
|
|
assert_se(bitmap_equal(b, b2));
|
|
|
|
assert_se(bitmap_set(b, 0) == 0);
|
|
assert_se(bitmap_set(b2, 0) == 0);
|
|
assert_se(bitmap_equal(b, b2));
|
|
|
|
return 0;
|
|
}
|