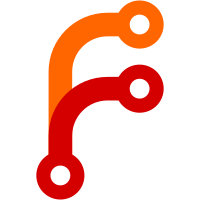
This primarily changes to things: 1. Ellipsation to 0, 1 or 2 characters is now supported. Previously we'd hit an assert if the new lengths was < 3, this is now permitted. The result strings won't show too much info still of course, but the code becomes a bit more generic and robust to use. 2. If a UTF-8 mode is disabled and the input string is pure ASCII, then "..." is used for ellipsation, otherwise (as before) "…". This means on a pure-ASCII system we should remain pure-ASCII, matching behaviour otherwise exposed with special_glyph() and friends. Note that we'll use "…" for ellipsiation as soon as either the locale settings indicate an UTF-8 mode or the input string already contains non-ASCII unicode characters. Testing for these special cases is improved.
58 lines
2.9 KiB
C
58 lines
2.9 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2013 Shawn Landden
|
|
***/
|
|
|
|
#include <stdio.h>
|
|
|
|
#include "alloc-util.h"
|
|
#include "def.h"
|
|
#include "string-util.h"
|
|
#include "terminal-util.h"
|
|
#include "util.h"
|
|
|
|
static void test_one(const char *p) {
|
|
_cleanup_free_ char *t;
|
|
t = ellipsize(p, columns(), 70);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, columns(), 0);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, columns(), 100);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 0, 50);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 1, 50);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 2, 50);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 3, 50);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 4, 50);
|
|
puts(t);
|
|
free(t);
|
|
t = ellipsize(p, 5, 50);
|
|
puts(t);
|
|
}
|
|
|
|
int main(int argc, char *argv[]) {
|
|
test_one(DIGITS LETTERS DIGITS LETTERS);
|
|
test_one("한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어한국어");
|
|
test_one("-日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国日本国");
|
|
test_one("中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国中国-中国中国中国中国中国中国中国中国中国中国中国中国中国");
|
|
test_one("sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd sÿstëmd");
|
|
test_one("🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮🐮");
|
|
test_one("Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.");
|
|
test_one("shórt");
|
|
|
|
return 0;
|
|
}
|