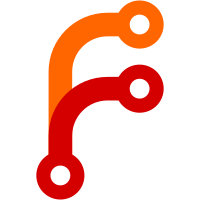
Files which are installed as-is (any .service and other unit files, .conf files, .policy files, etc), are left as is. My assumption is that SPDX identifiers are not yet that well known, so it's better to retain the extended header to avoid any doubt. I also kept any copyright lines. We can probably remove them, but it'd nice to obtain explicit acks from all involved authors before doing that.
60 lines
1.8 KiB
C
60 lines
1.8 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2010 Lennart Poettering
|
|
***/
|
|
|
|
#include <inttypes.h>
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
#include <sys/types.h>
|
|
#include <uchar.h>
|
|
|
|
#include "string-util.h"
|
|
#include "missing.h"
|
|
|
|
/* What characters are special in the shell? */
|
|
/* must be escaped outside and inside double-quotes */
|
|
#define SHELL_NEED_ESCAPE "\"\\`$"
|
|
|
|
/* Those that can be escaped or double-quoted.
|
|
*
|
|
* Stricly speaking, ! does not need to be escaped, except in interactive
|
|
* mode, but let's be extra nice to the user and quote ! in case this
|
|
* output is ever used in interactive mode. */
|
|
#define SHELL_NEED_QUOTES SHELL_NEED_ESCAPE GLOB_CHARS "'()<>|&;!"
|
|
|
|
/* Note that we assume control characters would need to be escaped too in
|
|
* addition to the "special" characters listed here, if they appear in the
|
|
* string. Current users disallow control characters. Also '"' shall not
|
|
* be escaped.
|
|
*/
|
|
#define SHELL_NEED_ESCAPE_POSIX "\\\'"
|
|
|
|
typedef enum UnescapeFlags {
|
|
UNESCAPE_RELAX = 1,
|
|
} UnescapeFlags;
|
|
|
|
typedef enum EscapeStyle {
|
|
ESCAPE_BACKSLASH = 1,
|
|
ESCAPE_POSIX = 2,
|
|
} EscapeStyle;
|
|
|
|
char *cescape(const char *s);
|
|
char *cescape_length(const char *s, size_t n);
|
|
size_t cescape_char(char c, char *buf);
|
|
|
|
int cunescape(const char *s, UnescapeFlags flags, char **ret);
|
|
int cunescape_length(const char *s, size_t length, UnescapeFlags flags, char **ret);
|
|
int cunescape_length_with_prefix(const char *s, size_t length, const char *prefix, UnescapeFlags flags, char **ret);
|
|
int cunescape_one(const char *p, size_t length, char32_t *ret, bool *eight_bit);
|
|
|
|
char *xescape(const char *s, const char *bad);
|
|
char *octescape(const char *s, size_t len);
|
|
|
|
char *shell_escape(const char *s, const char *bad);
|
|
char* shell_maybe_quote(const char *s, EscapeStyle style);
|