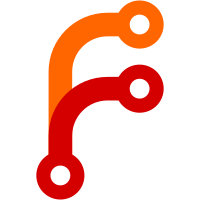
This beefs up the READ_FULL_FILE_CONNECT_SOCKET logic of read_full_file_full() a bit: when used a sender socket name may be specified. If specified as NULL behaviour is as before: the client socket name is picked by the kernel. But if specified as non-NULL the client can pick a socket name to use when connecting. This is useful to communicate a minimal amount of metainformation from client to server, outside of the transport payload. Specifically, these beefs up the service credential logic to pass an abstract AF_UNIX socket name as client socket name when connecting via READ_FULL_FILE_CONNECT_SOCKET, that includes the requesting unit name and the eventual credential name. This allows servers implementing the trivial credential socket logic to distinguish clients: via a simple getpeername() it can be determined which unit is requesting a credential, and which credential specifically. Example: with this patch in place, in a unit file "waldo.service" a configuration line like the following: LoadCredential=foo:/run/quux/creds.sock will result in a connection to the AF_UNIX socket /run/quux/creds.sock, originating from an abstract namespace AF_UNIX socket: @$RANDOM/unit/waldo.service/foo (The $RANDOM is replaced by some randomized string. This is included in the socket name order to avoid namespace squatting issues: the abstract socket namespace is open to unprivileged users after all, and care needs to be taken not to use guessable names) The services listening on the /run/quux/creds.sock socket may thus easily retrieve the name of the unit the credential is requested for plus the credential name, via a simpler getpeername(), discarding the random preifx and the /unit/ string. This logic uses "/" as separator between the fields, since both unit names and credential names appear in the file system, and thus are designed to use "/" as outer separators. Given that it's a good safe choice to use as separators here, too avoid any conflicts. This is a minimal patch only: the new logic is used only for the unit file credential logic. For other places where we use READ_FULL_FILE_CONNECT_SOCKET it is probably a good idea to use this scheme too, but this should be done carefully in later patches, since the socket names become API that way, and we should determine the right amount of info to pass over.
124 lines
5.2 KiB
C
124 lines
5.2 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
#include <dirent.h>
|
|
#include <stdbool.h>
|
|
#include <stddef.h>
|
|
#include <stdio.h>
|
|
#include <sys/stat.h>
|
|
#include <sys/fcntl.h>
|
|
#include <sys/types.h>
|
|
|
|
#include "macro.h"
|
|
#include "time-util.h"
|
|
|
|
#define LONG_LINE_MAX (1U*1024U*1024U)
|
|
|
|
typedef enum {
|
|
WRITE_STRING_FILE_CREATE = 1 << 0,
|
|
WRITE_STRING_FILE_TRUNCATE = 1 << 1,
|
|
WRITE_STRING_FILE_ATOMIC = 1 << 2,
|
|
WRITE_STRING_FILE_AVOID_NEWLINE = 1 << 3,
|
|
WRITE_STRING_FILE_VERIFY_ON_FAILURE = 1 << 4,
|
|
WRITE_STRING_FILE_SYNC = 1 << 5,
|
|
WRITE_STRING_FILE_DISABLE_BUFFER = 1 << 6,
|
|
WRITE_STRING_FILE_NOFOLLOW = 1 << 7,
|
|
WRITE_STRING_FILE_MKDIR_0755 = 1 << 8,
|
|
WRITE_STRING_FILE_MODE_0600 = 1 << 9,
|
|
|
|
/* And before you wonder, why write_string_file_atomic_label_ts() is a separate function instead of just one
|
|
more flag here: it's about linking: we don't want to pull -lselinux into all users of write_string_file()
|
|
and friends. */
|
|
|
|
} WriteStringFileFlags;
|
|
|
|
typedef enum {
|
|
READ_FULL_FILE_SECURE = 1 << 0, /* erase any buffers we employ internally, after use */
|
|
READ_FULL_FILE_UNBASE64 = 1 << 1, /* base64 decode what we read */
|
|
READ_FULL_FILE_UNHEX = 1 << 2, /* hex decode what we read */
|
|
READ_FULL_FILE_WARN_WORLD_READABLE = 1 << 3, /* if regular file, log at LOG_WARNING level if access mode above 0700 */
|
|
READ_FULL_FILE_CONNECT_SOCKET = 1 << 4, /* if socket inode, connect to it and read off it */
|
|
} ReadFullFileFlags;
|
|
|
|
int fopen_unlocked(const char *path, const char *options, FILE **ret);
|
|
int fdopen_unlocked(int fd, const char *options, FILE **ret);
|
|
int take_fdopen_unlocked(int *fd, const char *options, FILE **ret);
|
|
FILE* take_fdopen(int *fd, const char *options);
|
|
DIR* take_fdopendir(int *dfd);
|
|
FILE* open_memstream_unlocked(char **ptr, size_t *sizeloc);
|
|
FILE* fmemopen_unlocked(void *buf, size_t size, const char *mode);
|
|
|
|
int write_string_stream_ts(FILE *f, const char *line, WriteStringFileFlags flags, struct timespec *ts);
|
|
static inline int write_string_stream(FILE *f, const char *line, WriteStringFileFlags flags) {
|
|
return write_string_stream_ts(f, line, flags, NULL);
|
|
}
|
|
int write_string_file_ts(const char *fn, const char *line, WriteStringFileFlags flags, struct timespec *ts);
|
|
static inline int write_string_file(const char *fn, const char *line, WriteStringFileFlags flags) {
|
|
return write_string_file_ts(fn, line, flags, NULL);
|
|
}
|
|
|
|
int write_string_filef(const char *fn, WriteStringFileFlags flags, const char *format, ...) _printf_(3, 4);
|
|
|
|
int read_one_line_file(const char *filename, char **line);
|
|
int read_full_file_full(int dir_fd, const char *filename, ReadFullFileFlags flags, const char *bind_name, char **contents, size_t *size);
|
|
static inline int read_full_file(const char *filename, char **contents, size_t *size) {
|
|
return read_full_file_full(AT_FDCWD, filename, 0, NULL, contents, size);
|
|
}
|
|
int read_full_virtual_file(const char *filename, char **ret_contents, size_t *ret_size);
|
|
int read_full_stream_full(FILE *f, const char *filename, ReadFullFileFlags flags, char **contents, size_t *size);
|
|
static inline int read_full_stream(FILE *f, char **contents, size_t *size) {
|
|
return read_full_stream_full(f, NULL, 0, contents, size);
|
|
}
|
|
|
|
int verify_file(const char *fn, const char *blob, bool accept_extra_nl);
|
|
|
|
int executable_is_script(const char *path, char **interpreter);
|
|
|
|
int get_proc_field(const char *filename, const char *pattern, const char *terminator, char **field);
|
|
|
|
DIR *xopendirat(int dirfd, const char *name, int flags);
|
|
int xfopenat(int dir_fd, const char *path, const char *mode, int flags, FILE **ret);
|
|
|
|
int search_and_fopen(const char *path, const char *mode, const char *root, const char **search, FILE **_f);
|
|
int search_and_fopen_nulstr(const char *path, const char *mode, const char *root, const char *search, FILE **_f);
|
|
|
|
int chase_symlinks_and_fopen_unlocked(
|
|
const char *path,
|
|
const char *root,
|
|
unsigned chase_flags,
|
|
const char *open_flags,
|
|
FILE **ret_file,
|
|
char **ret_path);
|
|
|
|
int fflush_and_check(FILE *f);
|
|
int fflush_sync_and_check(FILE *f);
|
|
|
|
int write_timestamp_file_atomic(const char *fn, usec_t n);
|
|
int read_timestamp_file(const char *fn, usec_t *ret);
|
|
|
|
int fputs_with_space(FILE *f, const char *s, const char *separator, bool *space);
|
|
|
|
typedef enum ReadLineFlags {
|
|
READ_LINE_ONLY_NUL = 1 << 0,
|
|
READ_LINE_IS_A_TTY = 1 << 1,
|
|
READ_LINE_NOT_A_TTY = 1 << 2,
|
|
} ReadLineFlags;
|
|
|
|
int read_line_full(FILE *f, size_t limit, ReadLineFlags flags, char **ret);
|
|
|
|
static inline int read_line(FILE *f, size_t limit, char **ret) {
|
|
return read_line_full(f, limit, 0, ret);
|
|
}
|
|
|
|
static inline int read_nul_string(FILE *f, size_t limit, char **ret) {
|
|
return read_line_full(f, limit, READ_LINE_ONLY_NUL, ret);
|
|
}
|
|
|
|
int safe_fgetc(FILE *f, char *ret);
|
|
|
|
int warn_file_is_world_accessible(const char *filename, struct stat *st, const char *unit, unsigned line);
|
|
|
|
int sync_rights(int from, int to);
|
|
|
|
int rename_and_apply_smack_floor_label(const char *temp_path, const char *dest_path);
|