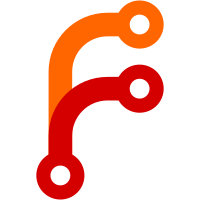
Files which are installed as-is (any .service and other unit files, .conf files, .policy files, etc), are left as is. My assumption is that SPDX identifiers are not yet that well known, so it's better to retain the extended header to avoid any doubt. I also kept any copyright lines. We can probably remove them, but it'd nice to obtain explicit acks from all involved authors before doing that.
81 lines
1.9 KiB
C
81 lines
1.9 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2014 David Herrmann <dh.herrmann@gmail.com>
|
|
***/
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
#include <sys/types.h>
|
|
|
|
#include "macro.h"
|
|
|
|
/* See source file for an API description. */
|
|
|
|
typedef struct Barrier Barrier;
|
|
|
|
enum {
|
|
BARRIER_SINGLE = 1LL,
|
|
BARRIER_ABORTION = INT64_MAX,
|
|
|
|
/* bias values to store state; keep @WE < @THEY < @I */
|
|
BARRIER_BIAS = INT64_MIN,
|
|
BARRIER_WE_ABORTED = BARRIER_BIAS + 1LL,
|
|
BARRIER_THEY_ABORTED = BARRIER_BIAS + 2LL,
|
|
BARRIER_I_ABORTED = BARRIER_BIAS + 3LL,
|
|
};
|
|
|
|
enum {
|
|
BARRIER_PARENT,
|
|
BARRIER_CHILD,
|
|
};
|
|
|
|
struct Barrier {
|
|
int me;
|
|
int them;
|
|
int pipe[2];
|
|
int64_t barriers;
|
|
};
|
|
|
|
#define BARRIER_NULL {-1, -1, {-1, -1}, 0}
|
|
|
|
int barrier_create(Barrier *obj);
|
|
void barrier_destroy(Barrier *b);
|
|
|
|
DEFINE_TRIVIAL_CLEANUP_FUNC(Barrier*, barrier_destroy);
|
|
|
|
void barrier_set_role(Barrier *b, unsigned int role);
|
|
|
|
bool barrier_place(Barrier *b);
|
|
bool barrier_abort(Barrier *b);
|
|
|
|
bool barrier_wait_next(Barrier *b);
|
|
bool barrier_wait_abortion(Barrier *b);
|
|
bool barrier_sync_next(Barrier *b);
|
|
bool barrier_sync(Barrier *b);
|
|
|
|
static inline bool barrier_i_aborted(Barrier *b) {
|
|
return IN_SET(b->barriers, BARRIER_I_ABORTED, BARRIER_WE_ABORTED);
|
|
}
|
|
|
|
static inline bool barrier_they_aborted(Barrier *b) {
|
|
return IN_SET(b->barriers, BARRIER_THEY_ABORTED, BARRIER_WE_ABORTED);
|
|
}
|
|
|
|
static inline bool barrier_we_aborted(Barrier *b) {
|
|
return b->barriers == BARRIER_WE_ABORTED;
|
|
}
|
|
|
|
static inline bool barrier_is_aborted(Barrier *b) {
|
|
return IN_SET(b->barriers,
|
|
BARRIER_I_ABORTED, BARRIER_THEY_ABORTED, BARRIER_WE_ABORTED);
|
|
}
|
|
|
|
static inline bool barrier_place_and_sync(Barrier *b) {
|
|
(void) barrier_place(b);
|
|
return barrier_sync(b);
|
|
}
|