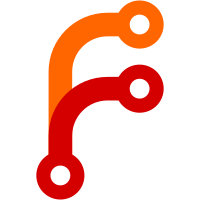
This was caused by derivations with 'allowSubstitutes = false'. Such derivations will be built locally. However, if there is another SubstitionGoal that has the output of the first derivation in its closure, then the path will be simultaneously built and substituted. There was a check to catch this situation (via pathIsLockedByMe()), but it no longer worked reliably because substitutions are now done in another thread. (Thus the comment 'It can't happen between here and the lockPaths() call below because we're not allowing multi-threading' was no longer valid.) The fix is to handle the path already being locked in both SubstitutionGoal and DerivationGoal.
43 lines
979 B
C++
43 lines
979 B
C++
#pragma once
|
|
|
|
#include "util.hh"
|
|
|
|
namespace nix {
|
|
|
|
/* Open (possibly create) a lock file and return the file descriptor.
|
|
-1 is returned if create is false and the lock could not be opened
|
|
because it doesn't exist. Any other error throws an exception. */
|
|
AutoCloseFD openLockFile(const Path & path, bool create);
|
|
|
|
/* Delete an open lock file. */
|
|
void deleteLockFile(const Path & path, int fd);
|
|
|
|
enum LockType { ltRead, ltWrite, ltNone };
|
|
|
|
bool lockFile(int fd, LockType lockType, bool wait);
|
|
|
|
MakeError(AlreadyLocked, Error);
|
|
|
|
class PathLocks
|
|
{
|
|
private:
|
|
typedef std::pair<int, Path> FDPair;
|
|
list<FDPair> fds;
|
|
bool deletePaths;
|
|
|
|
public:
|
|
PathLocks();
|
|
PathLocks(const PathSet & paths,
|
|
const string & waitMsg = "");
|
|
bool lockPaths(const PathSet & _paths,
|
|
const string & waitMsg = "",
|
|
bool wait = true);
|
|
~PathLocks();
|
|
void unlock();
|
|
void setDeletion(bool deletePaths);
|
|
};
|
|
|
|
bool pathIsLockedByMe(const Path & path);
|
|
|
|
}
|