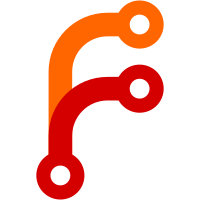
* libexpr: fix builtins.split example The example was previously indicating that multiple whitespaces would be collapsed into a single captured whitespace. That isn't true and was likely a mistake when being documented initially. * Fix segfault on unitilized list when printing value Since lists are just chunks of memory the individual elements in the list might be unitilized when a programming error happens within Nix. In this case the values are null-initialized (at least with Boehm GC) and we can avoid a nullptr deref when printing them. I ran into this issue while ensuring that new expression tests would show the actual value on an assertion failure. This is unlikely to cause any runtime performance regressions as printing values is not really in the hot path (unless the repl is the primary use case). * Add operator<< for ValueTypes * Add libexpr tests This introduces tests for libexpr that evalulate various trivial Nix language expressions and primop invocations that should be good smoke tests wheter or not the implementation is behaving as expected.
69 lines
1.7 KiB
C++
69 lines
1.7 KiB
C++
#include "libexprtests.hh"
|
|
#include "value-to-json.hh"
|
|
|
|
namespace nix {
|
|
// Testing the conversion to JSON
|
|
|
|
class JSONValueTest : public LibExprTest {
|
|
protected:
|
|
std::string getJSONValue(Value& value) {
|
|
std::stringstream ss;
|
|
PathSet ps;
|
|
printValueAsJSON(state, true, value, noPos, ss, ps);
|
|
return ss.str();
|
|
}
|
|
};
|
|
|
|
TEST_F(JSONValueTest, null) {
|
|
Value v;
|
|
v.mkNull();
|
|
ASSERT_EQ(getJSONValue(v), "null");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, BoolFalse) {
|
|
Value v;
|
|
v.mkBool(false);
|
|
ASSERT_EQ(getJSONValue(v),"false");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, BoolTrue) {
|
|
Value v;
|
|
v.mkBool(true);
|
|
ASSERT_EQ(getJSONValue(v), "true");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, IntPositive) {
|
|
Value v;
|
|
v.mkInt(100);
|
|
ASSERT_EQ(getJSONValue(v), "100");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, IntNegative) {
|
|
Value v;
|
|
v.mkInt(-100);
|
|
ASSERT_EQ(getJSONValue(v), "-100");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, String) {
|
|
Value v;
|
|
v.mkString("test");
|
|
ASSERT_EQ(getJSONValue(v), "\"test\"");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, StringQuotes) {
|
|
Value v;
|
|
|
|
v.mkString("test\"");
|
|
ASSERT_EQ(getJSONValue(v), "\"test\\\"\"");
|
|
}
|
|
|
|
// The dummy store doesn't support writing files. Fails with this exception message:
|
|
// C++ exception with description "error: operation 'addToStoreFromDump' is
|
|
// not supported by store 'dummy'" thrown in the test body.
|
|
TEST_F(JSONValueTest, DISABLED_Path) {
|
|
Value v;
|
|
v.mkPath("test");
|
|
ASSERT_EQ(getJSONValue(v), "\"/nix/store/g1w7hy3qg1w7hy3qg1w7hy3qg1w7hy3q-x\"");
|
|
}
|
|
} /* namespace nix */
|