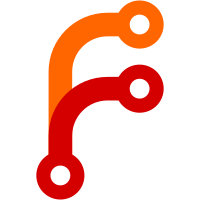
We upgrade to lowdown 0.8.0 [1] which contains a fix/improvement to a
behavior mentioned in this issue thread [2] where a big part of
lowdown's API would just call exit(1) on allocation errors since that
is a satisfying behavior for the lowdown binary.
Now lowdown_term_rndr returns 0 if an allocation error occurred which we
check for in libcmd/markdown.cc.
Also the extern "C" { } wrapper around lowdown.h has been removed as it
is not necessary.
[1]: 6ca7c855a0/versions.xml (L987-L1006)
[2]: https://github.com/kristapsdz/lowdown/issues/45#issuecomment-756681153
51 lines
1.4 KiB
C++
51 lines
1.4 KiB
C++
#include "markdown.hh"
|
|
#include "util.hh"
|
|
#include "finally.hh"
|
|
|
|
#include <sys/queue.h>
|
|
#include <lowdown.h>
|
|
|
|
namespace nix {
|
|
|
|
std::string renderMarkdownToTerminal(std::string_view markdown)
|
|
{
|
|
struct lowdown_opts opts {
|
|
.type = LOWDOWN_TERM,
|
|
.maxdepth = 20,
|
|
.cols = std::min(getWindowSize().second, (unsigned short) 80),
|
|
.hmargin = 0,
|
|
.vmargin = 0,
|
|
.feat = LOWDOWN_COMMONMARK | LOWDOWN_FENCED | LOWDOWN_DEFLIST | LOWDOWN_TABLES,
|
|
.oflags = 0,
|
|
};
|
|
|
|
auto doc = lowdown_doc_new(&opts);
|
|
if (!doc)
|
|
throw Error("cannot allocate Markdown document");
|
|
Finally freeDoc([&]() { lowdown_doc_free(doc); });
|
|
|
|
size_t maxn = 0;
|
|
auto node = lowdown_doc_parse(doc, &maxn, markdown.data(), markdown.size());
|
|
if (!node)
|
|
throw Error("cannot parse Markdown document");
|
|
Finally freeNode([&]() { lowdown_node_free(node); });
|
|
|
|
auto renderer = lowdown_term_new(&opts);
|
|
if (!renderer)
|
|
throw Error("cannot allocate Markdown renderer");
|
|
Finally freeRenderer([&]() { lowdown_term_free(renderer); });
|
|
|
|
auto buf = lowdown_buf_new(16384);
|
|
if (!buf)
|
|
throw Error("cannot allocate Markdown output buffer");
|
|
Finally freeBuffer([&]() { lowdown_buf_free(buf); });
|
|
|
|
int rndr_res = lowdown_term_rndr(buf, nullptr, renderer, node);
|
|
if (!rndr_res)
|
|
throw Error("allocation error while rendering Markdown");
|
|
|
|
return std::string(buf->data, buf->size);
|
|
}
|
|
|
|
}
|