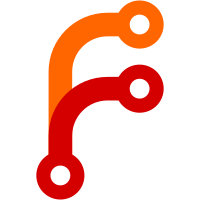
kernel 5.6 added support for a new flag for getrandom(): GRND_INSECURE. If we set it we can get some random data out of the kernel random pool, even if it is not yet initializated. This is great for us to initialize hash table seeds and such, where it is OK if they are crap initially. We used RDRAND for these cases so far, but RDRAND is only available on newer CPUs and some archs. Let's now use GRND_INSECURE for these cases as well, which means we won't needlessly delay boot anymore even on archs/CPUs that do not have RDRAND. Of course we never set this flag when generating crypto keys or uuids. Which makes it different from RDRAND for us (and is the reason I think we should keep explicit RDRAND support in): RDRAND we don't trust enough for crypto keys. But we do trust it enough for UUIDs.
41 lines
1.7 KiB
C
41 lines
1.7 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
#include <stdbool.h>
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
|
|
typedef enum RandomFlags {
|
|
RANDOM_EXTEND_WITH_PSEUDO = 1 << 0, /* If we can't get enough genuine randomness, but some, fill up the rest with pseudo-randomness */
|
|
RANDOM_BLOCK = 1 << 1, /* Rather block than return crap randomness (only if the kernel supports that) */
|
|
RANDOM_MAY_FAIL = 1 << 2, /* If we can't get any randomness at all, return early with -ENODATA */
|
|
RANDOM_ALLOW_RDRAND = 1 << 3, /* Allow usage of the CPU RNG */
|
|
RANDOM_ALLOW_INSECURE = 1 << 4, /* Allow usage of GRND_INSECURE flag to kernel's getrandom() API */
|
|
} RandomFlags;
|
|
|
|
int genuine_random_bytes(void *p, size_t n, RandomFlags flags); /* returns "genuine" randomness, optionally filled up with pseudo random, if not enough is available */
|
|
void pseudo_random_bytes(void *p, size_t n); /* returns only pseudo-randommess (but possibly seeded from something better) */
|
|
void random_bytes(void *p, size_t n); /* returns genuine randomness if cheaply available, and pseudo randomness if not. */
|
|
|
|
void initialize_srand(void);
|
|
|
|
static inline uint64_t random_u64(void) {
|
|
uint64_t u;
|
|
random_bytes(&u, sizeof(u));
|
|
return u;
|
|
}
|
|
|
|
static inline uint32_t random_u32(void) {
|
|
uint32_t u;
|
|
random_bytes(&u, sizeof(u));
|
|
return u;
|
|
}
|
|
|
|
int rdrand(unsigned long *ret);
|
|
|
|
/* Some limits on the pool sizes when we deal with the kernel random pool */
|
|
#define RANDOM_POOL_SIZE_MIN 512U
|
|
#define RANDOM_POOL_SIZE_MAX (10U*1024U*1024U)
|
|
|
|
size_t random_pool_size(void);
|