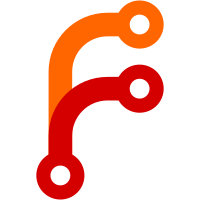
Don't try to merge devices that have been created via dependencies when they appear in the system and can be recognized as the same. Instead, simply continue to maintain them independently of each other, however with the same state cycle. Why? Because otherwise we'd have a hard time to seperate the dependencies after the devices are unplugged again and we hence cannot be sure anymore that next time the device is plugged in it will carry the same names. Example: if one depndency refers to dev-sda.device and another one to dev-by-id-xxxyyy.device we only learn at time of plug in of the device that it is actually the same device that was ment. In the moment the device is unplugged again we won't know anymore their relation to each other and the next time the harddisk is plugged it might even appear as dev-by-id-xxxyyy.device and dev-sdb.service. To ensure the dependencies continue to have the meaning they were intended to have let's hence keep the .device objects seperate all the time, even when they are plugged in. This patch also introduces a new Following= property which points from the various .device units of a specific device to the main .device unit for it. This can be used by the client side to figure out the relation of the .device units to each other and even filter units from display.
166 lines
4.7 KiB
C
166 lines
4.7 KiB
C
/*-*- Mode: C; c-basic-offset: 8 -*-*/
|
|
|
|
#ifndef fooservicehfoo
|
|
#define fooservicehfoo
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2010 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation; either version 2 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
typedef struct Service Service;
|
|
|
|
#include "unit.h"
|
|
#include "ratelimit.h"
|
|
|
|
typedef enum ServiceState {
|
|
SERVICE_DEAD,
|
|
SERVICE_START_PRE,
|
|
SERVICE_START,
|
|
SERVICE_START_POST,
|
|
SERVICE_RUNNING,
|
|
SERVICE_EXITED, /* Nothing is running anymore, but ValidNoProcess is true, ehnce this is OK */
|
|
SERVICE_RELOAD,
|
|
SERVICE_STOP, /* No STOP_PRE state, instead just register multiple STOP executables */
|
|
SERVICE_STOP_SIGTERM,
|
|
SERVICE_STOP_SIGKILL,
|
|
SERVICE_STOP_POST,
|
|
SERVICE_FINAL_SIGTERM, /* In case the STOP_POST executable hangs, we shoot that down, too */
|
|
SERVICE_FINAL_SIGKILL,
|
|
SERVICE_MAINTENANCE,
|
|
SERVICE_AUTO_RESTART,
|
|
_SERVICE_STATE_MAX,
|
|
_SERVICE_STATE_INVALID = -1
|
|
} ServiceState;
|
|
|
|
typedef enum ServiceRestart {
|
|
SERVICE_ONCE,
|
|
SERVICE_RESTART_ON_SUCCESS,
|
|
SERVICE_RESTART_ALWAYS,
|
|
_SERVICE_RESTART_MAX,
|
|
_SERVICE_RESTART_INVALID = -1
|
|
} ServiceRestart;
|
|
|
|
typedef enum ServiceType {
|
|
SERVICE_SIMPLE, /* we fork and go on right-away (i.e. modern socket activated daemons) */
|
|
SERVICE_FORKING, /* forks by itself (i.e. traditional daemons) */
|
|
SERVICE_FINISH, /* we fork and wait until the program finishes (i.e. programs like fsck which run and need to finish before we continue) */
|
|
SERVICE_DBUS, /* we fork and wait until a specific D-Bus name appears on the bus */
|
|
SERVICE_NOTIFY, /* we fork and wait until a daemon sends us a ready message with sd_notify() */
|
|
_SERVICE_TYPE_MAX,
|
|
_SERVICE_TYPE_INVALID = -1
|
|
} ServiceType;
|
|
|
|
typedef enum ServiceExecCommand {
|
|
SERVICE_EXEC_START_PRE,
|
|
SERVICE_EXEC_START,
|
|
SERVICE_EXEC_START_POST,
|
|
SERVICE_EXEC_RELOAD,
|
|
SERVICE_EXEC_STOP,
|
|
SERVICE_EXEC_STOP_POST,
|
|
_SERVICE_EXEC_COMMAND_MAX,
|
|
_SERVICE_EXEC_COMMAND_INVALID = -1
|
|
} ServiceExecCommand;
|
|
|
|
typedef enum NotifyAccess {
|
|
NOTIFY_NONE,
|
|
NOTIFY_ALL,
|
|
NOTIFY_MAIN,
|
|
_NOTIFY_ACCESS_MAX,
|
|
_NOTIFY_ACCESS_INVALID = -1
|
|
} NotifyAccess;
|
|
|
|
struct Service {
|
|
Meta meta;
|
|
|
|
ServiceType type;
|
|
ServiceRestart restart;
|
|
|
|
/* If set we'll read the main daemon PID from this file */
|
|
char *pid_file;
|
|
|
|
usec_t restart_usec;
|
|
usec_t timeout_usec;
|
|
|
|
ExecCommand* exec_command[_SERVICE_EXEC_COMMAND_MAX];
|
|
ExecContext exec_context;
|
|
|
|
ServiceState state, deserialized_state;
|
|
|
|
ExecStatus main_exec_status;
|
|
|
|
ExecCommand *control_command;
|
|
ServiceExecCommand control_command_id;
|
|
pid_t main_pid, control_pid;
|
|
|
|
bool permissions_start_only;
|
|
bool root_directory_start_only;
|
|
bool valid_no_process;
|
|
|
|
bool main_pid_known:1;
|
|
|
|
/* If we shut down, remember why */
|
|
bool failure:1;
|
|
|
|
bool bus_name_good:1;
|
|
|
|
bool allow_restart:1;
|
|
|
|
bool got_socket_fd:1;
|
|
|
|
bool sysv_has_lsb:1;
|
|
|
|
int socket_fd;
|
|
int sysv_start_priority;
|
|
|
|
char *sysv_path;
|
|
char *sysv_runlevels;
|
|
|
|
char *bus_name;
|
|
|
|
char *status_text;
|
|
|
|
RateLimit ratelimit;
|
|
|
|
struct Socket *socket;
|
|
|
|
Watch timer_watch;
|
|
|
|
NotifyAccess notify_access;
|
|
};
|
|
|
|
extern const UnitVTable service_vtable;
|
|
|
|
int service_set_socket_fd(Service *s, int fd, struct Socket *socket);
|
|
|
|
const char* service_state_to_string(ServiceState i);
|
|
ServiceState service_state_from_string(const char *s);
|
|
|
|
const char* service_restart_to_string(ServiceRestart i);
|
|
ServiceRestart service_restart_from_string(const char *s);
|
|
|
|
const char* service_type_to_string(ServiceType i);
|
|
ServiceType service_type_from_string(const char *s);
|
|
|
|
const char* service_exec_command_to_string(ServiceExecCommand i);
|
|
ServiceExecCommand service_exec_command_from_string(const char *s);
|
|
|
|
const char* notify_access_to_string(NotifyAccess i);
|
|
NotifyAccess notify_access_from_string(const char *s);
|
|
|
|
#endif
|