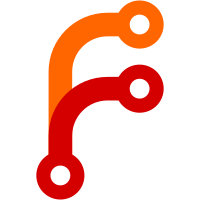
v246 is long released. Hence the new scheme should be named v247. (Interesting, how we pretty systematically for the last releases changed the scheme only every second release)
70 lines
2.1 KiB
C
70 lines
2.1 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
|
|
#include "alloc-util.h"
|
|
#include "netif-naming-scheme.h"
|
|
#include "proc-cmdline.h"
|
|
#include "string-util.h"
|
|
|
|
static const NamingScheme naming_schemes[] = {
|
|
{ "v238", NAMING_V238 },
|
|
{ "v239", NAMING_V239 },
|
|
{ "v240", NAMING_V240 },
|
|
{ "v241", NAMING_V241 },
|
|
{ "v243", NAMING_V243 },
|
|
{ "v245", NAMING_V245 },
|
|
{ "v247", NAMING_V247 },
|
|
/* … add more schemes here, as the logic to name devices is updated … */
|
|
};
|
|
|
|
static const NamingScheme* naming_scheme_from_name(const char *name) {
|
|
size_t i;
|
|
|
|
if (streq(name, "latest"))
|
|
return naming_schemes + ELEMENTSOF(naming_schemes) - 1;
|
|
|
|
for (i = 0; i < ELEMENTSOF(naming_schemes); i++)
|
|
if (streq(naming_schemes[i].name, name))
|
|
return naming_schemes + i;
|
|
|
|
return NULL;
|
|
}
|
|
|
|
const NamingScheme* naming_scheme(void) {
|
|
static const NamingScheme *cache = NULL;
|
|
_cleanup_free_ char *buffer = NULL;
|
|
const char *e, *k;
|
|
|
|
if (cache)
|
|
return cache;
|
|
|
|
/* Acquire setting from the kernel command line */
|
|
(void) proc_cmdline_get_key("net.naming-scheme", 0, &buffer);
|
|
|
|
/* Also acquire it from an env var */
|
|
e = getenv("NET_NAMING_SCHEME");
|
|
if (e) {
|
|
if (*e == ':') {
|
|
/* If prefixed with ':' the kernel cmdline takes precedence */
|
|
k = buffer ?: e + 1;
|
|
} else
|
|
k = e; /* Otherwise the env var takes precedence */
|
|
} else
|
|
k = buffer;
|
|
|
|
if (k) {
|
|
cache = naming_scheme_from_name(k);
|
|
if (cache) {
|
|
log_info("Using interface naming scheme '%s'.", cache->name);
|
|
return cache;
|
|
}
|
|
|
|
log_warning("Unknown interface naming scheme '%s' requested, ignoring.", k);
|
|
}
|
|
|
|
cache = naming_scheme_from_name(DEFAULT_NET_NAMING_SCHEME);
|
|
assert(cache);
|
|
log_info("Using default interface naming scheme '%s'.", cache->name);
|
|
|
|
return cache;
|
|
}
|