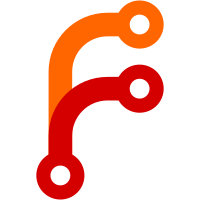
This implements RFC 5155, Section 8.8 and RFC 4035, Section 5.3.4: When we receive a response with an RRset generated from a wildcard we need to look for one NSEC/NSEC3 RR that proves that there's no explicit RR around before we accept the wildcard RRset as response. This patch does a couple of things: the validation calls will now identify wildcard signatures for us, and let us know the RRSIG used (so that the RRSIG's signer field let's us know what the wildcard was that generate the entry). Moreover, when iterating trough the RRsets of a response we now employ three phases instead of just two. a) in the first phase we only look for DNSKEYs RRs b) in the second phase we only look for NSEC RRs c) in the third phase we look for all kinds of RRs Phase a) is necessary, since DNSKEYs "unlock" more signatures for us, hence we shouldn't assume a key is missing until all DNSKEY RRs have been processed. Phase b) is necessary since NSECs need to be validated before we can validate wildcard RRs due to the logic explained above. Phase c) validates everything else. This phase also handles RRsets that cannot be fully validated and removes them or lets the transaction fail.
90 lines
3.5 KiB
C
90 lines
3.5 KiB
C
/*-*- Mode: C; c-basic-offset: 8; indent-tabs-mode: nil -*-*/
|
|
|
|
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2015 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
typedef enum DnssecMode DnssecMode;
|
|
typedef enum DnssecResult DnssecResult;
|
|
|
|
#include "dns-domain.h"
|
|
#include "resolved-dns-answer.h"
|
|
#include "resolved-dns-rr.h"
|
|
|
|
enum DnssecResult {
|
|
/* These five are returned by dnssec_verify_rrset() */
|
|
DNSSEC_VALIDATED,
|
|
DNSSEC_VALIDATED_WILDCARD, /* Validated via a wildcard RRSIG, further NSEC/NSEC3 checks necessary */
|
|
DNSSEC_INVALID,
|
|
DNSSEC_SIGNATURE_EXPIRED,
|
|
DNSSEC_UNSUPPORTED_ALGORITHM,
|
|
|
|
/* These two are added by dnssec_verify_rrset_search() */
|
|
DNSSEC_NO_SIGNATURE,
|
|
DNSSEC_MISSING_KEY,
|
|
|
|
/* These two are added by the DnsTransaction logic */
|
|
DNSSEC_UNSIGNED,
|
|
DNSSEC_FAILED_AUXILIARY,
|
|
DNSSEC_NSEC_MISMATCH,
|
|
DNSSEC_INCOMPATIBLE_SERVER,
|
|
|
|
_DNSSEC_RESULT_MAX,
|
|
_DNSSEC_RESULT_INVALID = -1
|
|
};
|
|
|
|
#define DNSSEC_CANONICAL_HOSTNAME_MAX (DNS_HOSTNAME_MAX + 2)
|
|
|
|
/* The longest digest we'll ever generate, of all digest algorithms we support */
|
|
#define DNSSEC_HASH_SIZE_MAX (MAX(20, 32))
|
|
|
|
int dnssec_rrsig_match_dnskey(DnsResourceRecord *rrsig, DnsResourceRecord *dnskey, bool revoked_ok);
|
|
int dnssec_key_match_rrsig(const DnsResourceKey *key, DnsResourceRecord *rrsig);
|
|
|
|
int dnssec_verify_rrset(DnsAnswer *answer, const DnsResourceKey *key, DnsResourceRecord *rrsig, DnsResourceRecord *dnskey, usec_t realtime, DnssecResult *result);
|
|
int dnssec_verify_rrset_search(DnsAnswer *answer, const DnsResourceKey *key, DnsAnswer *validated_dnskeys, usec_t realtime, DnssecResult *result, DnsResourceRecord **rrsig);
|
|
|
|
int dnssec_verify_dnskey(DnsResourceRecord *dnskey, DnsResourceRecord *ds, bool mask_revoke);
|
|
int dnssec_verify_dnskey_search(DnsResourceRecord *dnskey, DnsAnswer *validated_ds);
|
|
|
|
int dnssec_has_rrsig(DnsAnswer *a, const DnsResourceKey *key);
|
|
|
|
uint16_t dnssec_keytag(DnsResourceRecord *dnskey, bool mask_revoke);
|
|
|
|
int dnssec_canonicalize(const char *n, char *buffer, size_t buffer_max);
|
|
|
|
int dnssec_nsec3_hash(DnsResourceRecord *nsec3, const char *name, void *ret);
|
|
|
|
typedef enum DnssecNsecResult {
|
|
DNSSEC_NSEC_NO_RR, /* No suitable NSEC/NSEC3 RR found */
|
|
DNSSEC_NSEC_CNAME, /* Didn't find what was asked for, but did find CNAME */
|
|
DNSSEC_NSEC_UNSUPPORTED_ALGORITHM,
|
|
DNSSEC_NSEC_NXDOMAIN,
|
|
DNSSEC_NSEC_NODATA,
|
|
DNSSEC_NSEC_FOUND,
|
|
DNSSEC_NSEC_OPTOUT,
|
|
} DnssecNsecResult;
|
|
|
|
int dnssec_nsec_test(DnsAnswer *answer, DnsResourceKey *key, DnssecNsecResult *result, bool *authenticated, uint32_t *ttl);
|
|
int dnssec_nsec_test_between(DnsAnswer *answer, const char *name, const char *zone, bool *authenticated);
|
|
|
|
const char* dnssec_result_to_string(DnssecResult m) _const_;
|
|
DnssecResult dnssec_result_from_string(const char *s) _pure_;
|