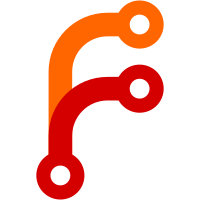
We should be careful with errno in cleanup functions, and not alter it under any circumstances. In the safe_close cleanup handlers we are already safe in that regard, but let's add similar protections on other cleanup handlers that invoke system calls. Why bother? Cleanup handlers insert code at function return in non-obvious ways. Hence, code that sets errno and returns should not be confused by us overrding the errno from a cleanup handler. This is a paranoia fix only, I am not aware where this actually mattered in real-life situations.
44 lines
1.4 KiB
C
44 lines
1.4 KiB
C
/* SPDX-License-Identifier: LGPL-2.1+ */
|
|
#pragma once
|
|
|
|
/***
|
|
This file is part of systemd.
|
|
|
|
Copyright 2015 Lennart Poettering
|
|
|
|
systemd is free software; you can redistribute it and/or modify it
|
|
under the terms of the GNU Lesser General Public License as published by
|
|
the Free Software Foundation; either version 2.1 of the License, or
|
|
(at your option) any later version.
|
|
|
|
systemd is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Lesser General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Lesser General Public License
|
|
along with systemd; If not, see <http://www.gnu.org/licenses/>.
|
|
***/
|
|
|
|
#include <sys/stat.h>
|
|
|
|
#include "util.h"
|
|
|
|
typedef enum RemoveFlags {
|
|
REMOVE_ONLY_DIRECTORIES = 1,
|
|
REMOVE_ROOT = 2,
|
|
REMOVE_PHYSICAL = 4, /* if not set, only removes files on tmpfs, never physical file systems */
|
|
REMOVE_SUBVOLUME = 8,
|
|
} RemoveFlags;
|
|
|
|
int rm_rf_children(int fd, RemoveFlags flags, struct stat *root_dev);
|
|
int rm_rf(const char *path, RemoveFlags flags);
|
|
|
|
/* Useful for usage with _cleanup_(), destroys a directory and frees the pointer */
|
|
static inline void rm_rf_physical_and_free(char *p) {
|
|
PROTECT_ERRNO;
|
|
(void) rm_rf(p, REMOVE_ROOT|REMOVE_PHYSICAL);
|
|
free(p);
|
|
}
|
|
DEFINE_TRIVIAL_CLEANUP_FUNC(char*, rm_rf_physical_and_free);
|