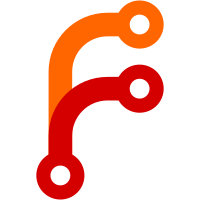
Here is the patch, that should prevent all of the known deadlocks with corrupt tdb databases we discovered. Thanks to Frank Steiner <fsteiner-mail@bio.ifi.lmu.de>, who tested all this endlessly with a NFS mounted /dev. The conclusion is, that udev will not work on filesystems without proper record locking, but we should prevent the endless loops anyway. This patch implements: o recovery from a corrupted udev database. udev will continue without database support now, instead of doing nothing. So the node should be generated in any case, remove will obviously not work for custom names. o added iteration limits to the tdb-code at the places we discovered endless loops. In the case tdb tries to find more than 100.000 entries with the same hash, we better give up :) o prevent a {all_partitions} loop caused by corrupt db data o log all tdb errors to syslog o switch sleep() to usleep() cause we want to use alarm()
97 lines
2.7 KiB
C
97 lines
2.7 KiB
C
/*
|
|
* udev.h
|
|
*
|
|
* Userspace devfs
|
|
*
|
|
* Copyright (C) 2003 Greg Kroah-Hartman <greg@kroah.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation version 2 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*
|
|
*/
|
|
|
|
#ifndef _UDEV_H_
|
|
#define _UDEV_H_
|
|
|
|
#include <sys/param.h>
|
|
#include "libsysfs/sysfs/libsysfs.h"
|
|
|
|
#define ALARM_TIMEOUT 30
|
|
#define WAIT_FOR_FILE_SECONDS 10
|
|
#define WAIT_FOR_FILE_RETRY_FREQ 10
|
|
#define COMMENT_CHARACTER '#'
|
|
|
|
#define NAME_SIZE 256
|
|
#define OWNER_SIZE 30
|
|
#define GROUP_SIZE 30
|
|
#define MODE_SIZE 8
|
|
|
|
#define ACTION_SIZE 32
|
|
#define DEVPATH_SIZE 256
|
|
#define SUBSYSTEM_SIZE 32
|
|
#define SEQNUM_SIZE 32
|
|
|
|
#define LINE_SIZE 256
|
|
|
|
#define FAKE 1
|
|
#define NOFAKE 0
|
|
|
|
/* length of public data to store in udevdb */
|
|
#define UDEVICE_LEN (offsetof(struct udevice, bus_id))
|
|
|
|
struct udevice {
|
|
char name[NAME_SIZE];
|
|
char owner[OWNER_SIZE];
|
|
char group[GROUP_SIZE];
|
|
char type;
|
|
int major;
|
|
int minor;
|
|
unsigned int mode; /* not mode_t due to conflicting definitions in different libcs */
|
|
char symlink[NAME_SIZE];
|
|
int partitions;
|
|
int config_line;
|
|
char config_file[NAME_SIZE];
|
|
long config_uptime;
|
|
|
|
/* private data that help us in building strings */
|
|
char bus_id[SYSFS_NAME_LEN];
|
|
char program_result[NAME_SIZE];
|
|
char kernel_number[NAME_SIZE];
|
|
char kernel_name[NAME_SIZE];
|
|
};
|
|
|
|
extern int udev_add_device(const char *path, const char *subsystem, int fake);
|
|
extern int udev_remove_device(const char *path, const char *subsystem);
|
|
extern void udev_init_config(void);
|
|
extern int udev_start(void);
|
|
extern int parse_get_pair(char **orig_string, char **left, char **right);
|
|
extern void dev_d_send(struct udevice *dev, const char *subsystem,
|
|
const char *devpath);
|
|
|
|
extern char **main_argv;
|
|
extern char **main_envp;
|
|
extern char sysfs_path[SYSFS_PATH_MAX];
|
|
extern char udev_root[PATH_MAX];
|
|
extern char udev_db_filename[PATH_MAX+NAME_MAX];
|
|
extern char udev_permissions_filename[PATH_MAX+NAME_MAX];
|
|
extern char udev_config_filename[PATH_MAX+NAME_MAX];
|
|
extern char udev_rules_filename[PATH_MAX+NAME_MAX];
|
|
extern char default_mode_str[MODE_SIZE];
|
|
extern char default_owner_str[OWNER_SIZE];
|
|
extern char default_group_str[GROUP_SIZE];
|
|
extern int udev_log;
|
|
extern int udev_sleep;
|
|
extern int udev_dev_d;
|
|
|
|
#endif
|