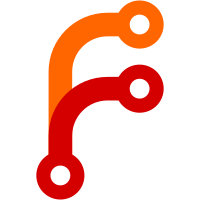
1998-04-07 20:32 Ulrich Drepper <drepper@cygnus.com> * wcsmbs/btowc.c: Fix dozends of bugs in untested code. * wcsmbs/mbrtowc.c: Likewise. * wcsmbs/mbsnrtowcs.c: Likewise. * wcsmbs/mbsrtowcs.c: Likewise. * wcsmbs/wcrtomb.c: Likewise. * wcsmbs/wcsnrtombs.c: Likewise. * wcsmbs/wcsrtombs.c: Likewise. * wcsmbs/wctob.c: Likewise. * iconv/gconv-simple.c (__gconv_transform_ascii_ucs4): Compute position of next output character correctly. (__gconv_transform_ucs4_ascii): Count used input bytes correctly. * stdio-common/vfprintf.c (vfprintf): Clear state before used. * stdlib/strtod.c: Don't use mbtowc. 1998-04-07 19:07 H.J. Lu <hjl@gnu.org> * libio/fileops.c (_IO_file_xsgetn): Call __underflow () when what we want is smaller than a buffer. 1998-04-07 18:14 Ulrich Drepper <drepper@cygnus.com> * elf/dl-support.c (_dl_important_hwcaps): Define dummy version of this function.
130 lines
3.6 KiB
C
130 lines
3.6 KiB
C
/* Copyright (C) 1996, 1997, 1998 Free Software Foundation, Inc.
|
|
This file is part of the GNU C Library.
|
|
Contributed by Ulrich Drepper <drepper@gnu.ai.mit.edu>, 1996.
|
|
|
|
The GNU C Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The GNU C Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the GNU C Library; see the file COPYING.LIB. If not,
|
|
write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA. */
|
|
|
|
#include <errno.h>
|
|
#include <gconv.h>
|
|
#include <string.h>
|
|
#include <wchar.h>
|
|
#include <wcsmbsload.h>
|
|
|
|
#include <assert.h>
|
|
|
|
#ifndef EILSEQ
|
|
# define EILSEQ EINVAL
|
|
#endif
|
|
|
|
|
|
/* This is the private state used if PS is NULL. */
|
|
static mbstate_t state;
|
|
|
|
size_t
|
|
__mbsrtowcs (dst, src, len, ps)
|
|
wchar_t *dst;
|
|
const char **src;
|
|
size_t len;
|
|
mbstate_t *ps;
|
|
{
|
|
struct gconv_step_data data;
|
|
size_t result = 0;
|
|
int status;
|
|
|
|
/* Tell where we want the result. */
|
|
data.is_last = 1;
|
|
data.statep = ps ?: &state;
|
|
|
|
/* Make sure we use the correct function. */
|
|
update_conversion_ptrs ();
|
|
|
|
/* We have to handle DST == NULL special. */
|
|
if (dst == NULL)
|
|
{
|
|
wchar_t buf[64]; /* Just an arbitrary size. */
|
|
size_t inbytes_in = strlen (*src) + 1;
|
|
size_t inbytes = inbytes_in;
|
|
const char *inbuf = *src;
|
|
size_t written;
|
|
|
|
data.outbuf = (char *) buf;
|
|
data.outbufsize = sizeof (buf);
|
|
do
|
|
{
|
|
inbuf += inbytes_in - inbytes;
|
|
inbytes_in = inbytes;
|
|
data.outbufavail = 0;
|
|
written = 0;
|
|
|
|
status = (*__wcsmbs_gconv_fcts.towc->fct) (__wcsmbs_gconv_fcts.towc,
|
|
&data, inbuf, &inbytes,
|
|
&written, 0);
|
|
result += written;
|
|
}
|
|
while (status == GCONV_FULL_OUTPUT);
|
|
|
|
if ((status == GCONV_OK || status == GCONV_EMPTY_INPUT)
|
|
&& buf[written - 1] == L'\0')
|
|
/* Don't count the NUL character in. */
|
|
--result;
|
|
}
|
|
else
|
|
{
|
|
/* This code is based on the safe assumption that all internal
|
|
multi-byte encodings use the NUL byte only to mark the end
|
|
of the string. */
|
|
size_t inbytes_in = __strnlen (*src, len * MB_CUR_MAX) + 1;
|
|
size_t inbytes = inbytes_in;
|
|
|
|
data.outbuf = (char *) dst;
|
|
data.outbufsize = len * sizeof (wchar_t);
|
|
data.outbufavail = 0;
|
|
|
|
status = (*__wcsmbs_gconv_fcts.towc->fct) (__wcsmbs_gconv_fcts.towc,
|
|
&data, *src, &inbytes,
|
|
&result, 0);
|
|
|
|
/* We have to determine whether the last character converted
|
|
is the NUL character. */
|
|
if ((status == GCONV_OK || status == GCONV_EMPTY_INPUT)
|
|
&& ((wchar_t *) dst)[result - 1] == L'\0')
|
|
{
|
|
assert (result > 0);
|
|
assert (mbsinit (data.statep));
|
|
*src = NULL;
|
|
--result;
|
|
}
|
|
else
|
|
*src += inbytes_in - inbytes;
|
|
}
|
|
|
|
/* There must not be any problems with the conversion but illegal input
|
|
characters. */
|
|
assert (status == GCONV_OK || status == GCONV_EMPTY_INPUT
|
|
|| status == GCONV_ILLEGAL_INPUT
|
|
|| status == GCONV_INCOMPLETE_INPUT || status == GCONV_FULL_OUTPUT);
|
|
|
|
if (status != GCONV_OK && status != GCONV_FULL_OUTPUT
|
|
&& status != GCONV_EMPTY_INPUT)
|
|
{
|
|
result = (size_t) -1;
|
|
__set_errno (EILSEQ);
|
|
}
|
|
|
|
return result;
|
|
}
|
|
weak_alias (__mbsrtowcs, mbsrtowcs)
|