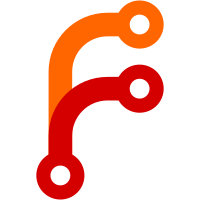
Replaced all imports of test-skeleton.c with support/test-driver.c. In some cases it was needed to adjust do_test to return int instead of static int since that is the method's signature expected by test-driver.c. Checked on x86_64. * string/test-string.h (TEST_FUNCTION): Use test_main instead of test_main (). (CMDLINE_PROCESS): Use function instead of defined macro. * debug/test-strcpy_chk.c: Import support/test-driver.c and also <suppport/support.h> to use set_fortify_handler(). * string/bug-envz1.c: Import support/test-driver.c instead of test-skeleton.c. * string/bug-strcoll2.c: Likewise. * string/bug-strtok1.c: Likewise. * string/stratcliff.c: Likewise. * string/test-ffs.c: Likewise. * string/test-memccpy.c: Likewise. * string/test-memchr.c: Likewise. * string/test-memcmp.c: Likewise. * string/test-memcpy.c: Likewise. * string/test-memmem.c: Likewise. * string/test-memmove.c: Likewise. * string/test-memrchr.c: Likewise. * string/test-memset.c: Likewise. * string/test-rawmemchr.c: Likewise. * string/test-strcasecmp.c: Likewise. * string/test-strcasestr.c: Likewise. * string/test-strcat.c: Likewise. * string/test-strchr.c: Likewise. * string/test-strcmp.c: Likewise. * string/test-strcpy.c: Likewise. * string/test-string.h: Likewise. * string/test-strlen.c: Likewise. * string/test-strncasecmp.c: Likewise. * string/test-strncat.c: Likewise. * string/test-strncmp.c: Likewise. * string/test-strncpy.c: Likewise. * string/test-strnlen.c: Likewise. * string/test-strpbrk.c: Likewise. * string/test-strrchr.c: Likewise. * string/test-strspn.c: Likewise. * string/test-strstr.c: Likewise. * string/tst-bswap.c: Likewise. * string/tst-cmp.c: Likewise. * string/tst-endian.c: Likewise. * string/tst-inlcall.c: Likewise. * string/tst-strcoll-overflow.c: Likewise. * string/tst-strfry.c: Likewise. * string/tst-strlen.c: Likewise. * string/tst-strtok.c: Likewise. * string/tst-strtok_r.c: Likewise. * string/tst-strxfrm.c: Likewise. * string/tst-strxfrm2.c: Likewise. * string/tst-svc.c: Likewise. * string/tst-svc2.c: Likewise.
58 lines
1.3 KiB
C
58 lines
1.3 KiB
C
/* Make sure we don't test the optimized inline functions if we want to
|
|
test the real implementation. */
|
|
#undef __USE_STRING_INLINES
|
|
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
|
|
int
|
|
do_test (void)
|
|
{
|
|
static const size_t lens[] = { 0, 1, 0, 2, 0, 1, 0, 3,
|
|
0, 1, 0, 2, 0, 1, 0, 4 };
|
|
char basebuf[24 + 32];
|
|
size_t base;
|
|
|
|
for (base = 0; base < 32; ++base)
|
|
{
|
|
char *buf = basebuf + base;
|
|
size_t words;
|
|
|
|
for (words = 0; words < 4; ++words)
|
|
{
|
|
size_t last;
|
|
memset (buf, 'a', words * 4);
|
|
|
|
for (last = 0; last < 16; ++last)
|
|
{
|
|
buf[words * 4 + 0] = (last & 1) != 0 ? 'b' : '\0';
|
|
buf[words * 4 + 1] = (last & 2) != 0 ? 'c' : '\0';
|
|
buf[words * 4 + 2] = (last & 4) != 0 ? 'd' : '\0';
|
|
buf[words * 4 + 3] = (last & 8) != 0 ? 'e' : '\0';
|
|
buf[words * 4 + 4] = '\0';
|
|
|
|
if (strlen (buf) != words * 4 + lens[last])
|
|
{
|
|
printf ("\
|
|
strlen failed for base=%Zu, words=%Zu, and last=%Zu (is %zd, expected %zd)\n",
|
|
base, words, last,
|
|
strlen (buf), words * 4 + lens[last]);
|
|
return 1;
|
|
}
|
|
|
|
if (strnlen (buf, -1) != words * 4 + lens[last])
|
|
{
|
|
printf ("\
|
|
strnlen failed for base=%Zu, words=%Zu, and last=%Zu (is %zd, expected %zd)\n",
|
|
base, words, last,
|
|
strnlen (buf, -1), words * 4 + lens[last]);
|
|
return 1;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
#include <support/test-driver.c>
|