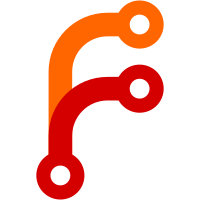
2002-02-07 Andreas Schwab <schwab@suse.de> * configure.in: Fix check for -zcombreloc. 2002-02-06 H.J. Lu <hjl@gnu.org> * config.h.in (HAVE_BUILTIN_MEMSET): New. * configure.in: Check if __builtin_memset really works. * elf/rtld.c (_dl_start): Check HAVE_BUILTIN_MEMSET instead of __GNUC_PREREQ (2, 96) before using __builtin_memset. 2002-02-06 Jakub Jelinek <jakub@redhat.com> * io/bug-ftw3.c (main): Don't try the test if root. 2002-02-06 Martin Schwidefsky <schwidefsky@de.ibm.com> * sysdeps/unix/sysv/linux/s390/brk.c (__brk): Correct inline assembly constraints. * sysdeps/unix/sysv/linux/s390/s390-32/bits/resource.h (RLIMIT_LOCKS): Add RLIMIT_LOCKS and adjust RLIMIT_NLIMITS. * sysdeps/unix/sysv/linux/s390/s390-64/bits/resource.h (RLIMIT_LOCKS): Likewise. * sysdeps/unix/sysv/linux/s390/s390-32/clone.S (clone): Make clone a weak alias for __clone. * sysdeps/unix/sysv/linux/s390/s390-64/clone.S (clone): Likewise. * sysdeps/unix/sysv/linux/s390/s390-32/profil-counter.h: Fix typo. * sysdeps/unix/sysv/linux/s390/s390-64/Makefile: Add framestate. * sysdeps/unix/sysv/linux/s390/s390-64/Versions: New file. * sysdeps/unix/sysv/linux/s390/s390-64/mmap.S (__mmap64): Make __mmap a weak alias for __mmap64. * sysdeps/mips/atomicity.h (exchange_and_add): Not use branch likely. * sysdeps/unix/sysv/linux/mips/sys/tas.h (_test_and_set): Likewise. * sysdeps/generic/dl-tls.c: Don't read TLS header if TLS is not needed.
70 lines
1.2 KiB
C
70 lines
1.2 KiB
C
#include <errno.h>
|
|
#include <ftw.h>
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
|
|
static int cb_called;
|
|
|
|
static int
|
|
cb (const char *fname, const struct stat *st, int flag)
|
|
{
|
|
printf ("%s %d\n", fname, flag);
|
|
cb_called = 1;
|
|
return 0;
|
|
}
|
|
|
|
int
|
|
main (void)
|
|
{
|
|
char tmp[] = "/tmp/ftwXXXXXX";
|
|
char tmp2[] = "/tmp/ftwXXXXXX/ftwXXXXXX";
|
|
char *dname;
|
|
char *dname2;
|
|
int r;
|
|
int e;
|
|
|
|
if (getuid () == 0)
|
|
{
|
|
puts ("this test needs to be run by ordinary user");
|
|
exit (0);
|
|
}
|
|
|
|
dname = mkdtemp (tmp);
|
|
if (dname == NULL)
|
|
{
|
|
printf ("mkdtemp: %m\n");
|
|
exit (1);
|
|
}
|
|
|
|
memcpy (tmp2, tmp, strlen (tmp));
|
|
dname2 = mkdtemp (tmp2);
|
|
if (dname2 == NULL)
|
|
{
|
|
printf ("mkdtemp: %m\n");
|
|
rmdir (dname);
|
|
exit (1);
|
|
}
|
|
|
|
if (chmod (dname, S_IWUSR|S_IWGRP|S_IWOTH) != 0)
|
|
{
|
|
printf ("chmod: %m\n");
|
|
rmdir (dname);
|
|
exit (1);
|
|
}
|
|
|
|
r = ftw (dname2, cb, 10);
|
|
e = errno;
|
|
printf ("r = %d", r);
|
|
if (r != 0)
|
|
printf (", errno = %d", errno);
|
|
puts ("");
|
|
|
|
chmod (dname, S_IRWXU|S_IRWXG|S_IRWXO);
|
|
rmdir (dname2);
|
|
rmdir (dname);
|
|
|
|
return (r != -1 && e == EACCES) || cb_called;
|
|
}
|