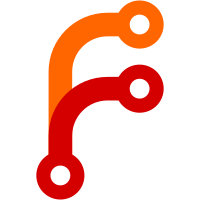
2003-12-02 David Mosberger <davidm@hpl.hp.com> * sysdeps/ia64/elf/initfini.c: Add unwind info. * sysdeps/ia64/dl-machine.h (elf_machine_matches_host): Mark with attribute "unused". (elf_machine_dynamic): Mark with attributes "unused" and "const". (elf_machine_runtime_setup): Likewise. * sysdeps/generic/dl-fptr.c (make_fptr_table): Mark with attribute "always_inline". * sysdeps/ia64/dl-machine.h (__ia64_init_bootstrap_fdesc_table): Likewise. * configure.in: Check whether compiler has libunwind support. * config.make.in (have-cc-with-libunwind): New variable. * config.h.in (HAVE_CC_WITH_LIBUNWIND): New macro. * Makeconfig (gnulib): If have-cc-withh-libunwind is "yes", also mention -lunwind. 003-11-12 David Mosberger <davidm@hpl.hp.com> * sysdeps/unix/sysv/linux/ia64/sysdep.h: Define DO_CALL_VIA_BREAK. Redefine DO_CALL to use vdso if supported, otherwise DO_CALL_VIA_BREAK. Likewise for DO_INLINE_SYSCALL. Make INTERNAL_SYSCALL use DO_INLINE_SYSCALL. * sysdeps/unix/sysv/linux/ia64/vfork.S: Use DO_CALL_VIA_BREAK() instead of DO_CALL(). * sysdeps/unix/sysv/linux/ia64/clone2.S: Use break directly instead of DO_CALL(). * sysdeps/unix/sysv/linux/ia64/brk.S (__curbrk): Restructure it to take advantage of DO_CALL() macro. * sysdeps/unix/sysv/linux/ia64/setcontext.S: Likewise. * sysdeps/unix/sysv/linux/ia64/getcontext.S: Likewise. * elf/rtld.c (dl_main): Restrict dl_sysinfo_dso check to first program header. On ia64, the check failed previously because there are two program headers. * sysdeps/generic/s_nexttowardf.c: Likewise. * math/bug-nexttoward.c: New file.
87 lines
2.3 KiB
C
87 lines
2.3 KiB
C
/* Single precision version of nexttoward.c.
|
|
Conversion to IEEE single float by Jakub Jelinek, jj@ultra.linux.cz. */
|
|
/*
|
|
* ====================================================
|
|
* Copyright (C) 1993 by Sun Microsystems, Inc. All rights reserved.
|
|
*
|
|
* Developed at SunPro, a Sun Microsystems, Inc. business.
|
|
* Permission to use, copy, modify, and distribute this
|
|
* software is freely granted, provided that this notice
|
|
* is preserved.
|
|
* ====================================================
|
|
*/
|
|
|
|
/* IEEE functions
|
|
* nexttowardf(x,y)
|
|
* return the next machine floating-point number of x in the
|
|
* direction toward y.
|
|
* This is for machines which use the same binary type for double and
|
|
* long double.
|
|
* Special cases:
|
|
*/
|
|
|
|
#include "math.h"
|
|
#include "math_private.h"
|
|
#include <float.h>
|
|
|
|
#ifdef __STDC__
|
|
float __nexttowardf(float x, long double y)
|
|
#else
|
|
float __nexttowardf(x,y)
|
|
float x;
|
|
long double y;
|
|
#endif
|
|
{
|
|
int32_t hx,hy,ix,iy;
|
|
u_int32_t ly;
|
|
|
|
GET_FLOAT_WORD(hx,x);
|
|
EXTRACT_WORDS(hy,ly,y);
|
|
ix = hx&0x7fffffff; /* |x| */
|
|
iy = hy&0x7fffffff; /* |y| */
|
|
|
|
if((ix>0x7f800000) || /* x is nan */
|
|
((iy>=0x7ff00000)&&((iy-0x7ff00000)|ly)!=0)) /* y is nan */
|
|
return x+y;
|
|
if((long double) x==y) return y; /* x=y, return y */
|
|
if(ix==0) { /* x == 0 */
|
|
float x2;
|
|
SET_FLOAT_WORD(x,(u_int32_t)(hy&0x80000000)|1);/* return +-minsub*/
|
|
x2 = x*x;
|
|
if(x2==x) return x2; else return x; /* raise underflow flag */
|
|
}
|
|
if(hx>=0) { /* x > 0 */
|
|
if(hy<0||(ix>>23)>(iy>>20)-0x380
|
|
|| ((ix>>23)==(iy>>20)-0x380
|
|
&& (ix&0x7fffff)>(((hy<<3)|(ly>>29))&0x7fffff))) /* x > y, x -= ulp */
|
|
hx -= 1;
|
|
else /* x < y, x += ulp */
|
|
hx += 1;
|
|
} else { /* x < 0 */
|
|
if(hy>=0||(ix>>23)>(iy>>20)-0x380
|
|
|| ((ix>>23)==(iy>>20)-0x380
|
|
&& (ix&0x7fffff)>(((hy<<3)|(ly>>29))&0x7fffff))) /* x < y, x -= ulp */
|
|
hx -= 1;
|
|
else /* x > y, x += ulp */
|
|
hx += 1;
|
|
}
|
|
hy = hx&0x7f800000;
|
|
if(hy>=0x7f800000) {
|
|
x = x+x; /* overflow */
|
|
if (FLT_EVAL_METHOD != 0)
|
|
/* Force conversion to float. */
|
|
asm ("" : "=m"(x) : "m"(x));
|
|
return x;
|
|
}
|
|
if(hy<0x00800000) { /* underflow */
|
|
float x2 = x*x;
|
|
if(x2!=x) { /* raise underflow flag */
|
|
SET_FLOAT_WORD(x2,hx);
|
|
return x2;
|
|
}
|
|
}
|
|
SET_FLOAT_WORD(x,hx);
|
|
return x;
|
|
}
|
|
weak_alias (__nexttowardf, nexttowardf)
|