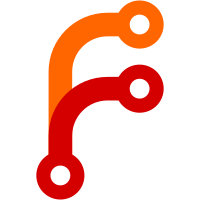
With static pie linking pointers in the tunables list need RELATIVE relocs since the absolute address is not known at link time. We want to avoid relocations so the static pie self relocation can be done after tunables are initialized. This is a simple fix that embeds the tunable strings into the tunable list instead of using pointers. It is possible to have a more compact representation of tunables with some additional complexity in the generator and tunable parser logic. Such optimization will be useful if the list of tunables grows. There is still an issue that tunables_strdup allocates and the failure handling code path is sufficiently complex that it can easily have RELATIVE relocations. It is possible to avoid the early allocation and only change environment variables in a setuid exe after relocations are processed. But that is a bigger change and early failure is fatal anyway so it is not as critical to fix right away. This is bug 27181. Reviewed-by: Adhemerval Zanella <adhemerval.zanella@linaro.org>
187 lines
4.5 KiB
Awk
187 lines
4.5 KiB
Awk
# Generate dl-tunable-list.h from dl-tunables.list
|
|
|
|
BEGIN {
|
|
min_of["STRING"]="0"
|
|
max_of["STRING"]="0"
|
|
min_of["INT_32"]="INT32_MIN"
|
|
max_of["INT_32"]="INT32_MAX"
|
|
min_of["UINT_64"]="0"
|
|
max_of["UINT_64"]="UINT64_MAX"
|
|
min_of["SIZE_T"]="0"
|
|
max_of["SIZE_T"]="SIZE_MAX"
|
|
tunable=""
|
|
ns=""
|
|
top_ns=""
|
|
max_name_len=0
|
|
max_alias_len=0
|
|
}
|
|
|
|
# Skip over blank lines and comments.
|
|
/^#/ {
|
|
next
|
|
}
|
|
|
|
/^[ \t]*$/ {
|
|
next
|
|
}
|
|
|
|
# Beginning of either a top namespace, tunable namespace or a tunable, decided
|
|
# on the current value of TUNABLE, NS or TOP_NS.
|
|
$2 == "{" {
|
|
if (top_ns == "") {
|
|
top_ns = $1
|
|
}
|
|
else if (ns == "") {
|
|
ns = $1
|
|
}
|
|
else if (tunable == "") {
|
|
tunable = $1
|
|
}
|
|
else {
|
|
printf ("Unexpected occurrence of '{': %s:%d\n", FILENAME, FNR)
|
|
exit 1
|
|
}
|
|
|
|
next
|
|
}
|
|
|
|
# End of either a top namespace, tunable namespace or a tunable.
|
|
$1 == "}" {
|
|
if (tunable != "") {
|
|
# Tunables definition ended, now fill in default attributes.
|
|
if (!types[top_ns,ns,tunable]) {
|
|
types[top_ns,ns,tunable] = "STRING"
|
|
}
|
|
if (!minvals[top_ns,ns,tunable]) {
|
|
minvals[top_ns,ns,tunable] = min_of[types[top_ns,ns,tunable]]
|
|
}
|
|
if (!maxvals[top_ns,ns,tunable]) {
|
|
maxvals[top_ns,ns,tunable] = max_of[types[top_ns,ns,tunable]]
|
|
}
|
|
if (!env_alias[top_ns,ns,tunable]) {
|
|
env_alias[top_ns,ns,tunable] = "{0}"
|
|
}
|
|
if (!security_level[top_ns,ns,tunable]) {
|
|
security_level[top_ns,ns,tunable] = "SXID_ERASE"
|
|
}
|
|
len = length(top_ns"."ns"."tunable)
|
|
if (len > max_name_len)
|
|
max_name_len = len
|
|
|
|
tunable = ""
|
|
}
|
|
else if (ns != "") {
|
|
ns = ""
|
|
}
|
|
else if (top_ns != "") {
|
|
top_ns = ""
|
|
}
|
|
else {
|
|
printf ("syntax error: extra }: %s:%d\n", FILENAME, FNR)
|
|
exit 1
|
|
}
|
|
next
|
|
}
|
|
|
|
# Everything else, which could either be a tunable without any attributes or a
|
|
# tunable attribute.
|
|
{
|
|
if (ns == "") {
|
|
printf("Line %d: Invalid tunable outside a namespace: %s\n", NR, $0)
|
|
exit 1
|
|
}
|
|
|
|
if (tunable == "") {
|
|
# We encountered a tunable without any attributes, so note it with a
|
|
# default.
|
|
types[top_ns,ns,$1] = "STRING"
|
|
next
|
|
}
|
|
|
|
# Otherwise, we have encountered a tunable attribute.
|
|
split($0, arr, ":")
|
|
attr = gensub(/^[ \t]+|[ \t]+$/, "", "g", arr[1])
|
|
val = gensub(/^[ \t]+|[ \t]+$/, "", "g", arr[2])
|
|
|
|
if (attr == "type") {
|
|
types[top_ns,ns,tunable] = val
|
|
}
|
|
else if (attr == "minval") {
|
|
minvals[top_ns,ns,tunable] = val
|
|
}
|
|
else if (attr == "maxval") {
|
|
maxvals[top_ns,ns,tunable] = val
|
|
}
|
|
else if (attr == "env_alias") {
|
|
env_alias[top_ns,ns,tunable] = sprintf("\"%s\"", val)
|
|
len = length(val)
|
|
if (len > max_alias_len)
|
|
max_alias_len = len
|
|
}
|
|
else if (attr == "security_level") {
|
|
if (val == "SXID_ERASE" || val == "SXID_IGNORE" || val == "NONE") {
|
|
security_level[top_ns,ns,tunable] = val
|
|
}
|
|
else {
|
|
printf("Line %d: Invalid value (%s) for security_level: %s, ", NR, val,
|
|
$0)
|
|
print("Allowed values are 'SXID_ERASE', 'SXID_IGNORE', or 'NONE'")
|
|
exit 1
|
|
}
|
|
}
|
|
else if (attr == "default") {
|
|
if (types[top_ns,ns,tunable] == "STRING") {
|
|
default_val[top_ns,ns,tunable] = sprintf(".strval = \"%s\"", val);
|
|
}
|
|
else {
|
|
default_val[top_ns,ns,tunable] = sprintf(".numval = %s", val)
|
|
}
|
|
}
|
|
}
|
|
|
|
END {
|
|
if (ns != "") {
|
|
print "Unterminated namespace. Is a closing brace missing?"
|
|
exit 1
|
|
}
|
|
|
|
print "/* AUTOGENERATED by gen-tunables.awk. */"
|
|
print "#ifndef _TUNABLES_H_"
|
|
print "# error \"Do not include this file directly.\""
|
|
print "# error \"Include tunables.h instead.\""
|
|
print "#endif"
|
|
print "#include <dl-procinfo.h>\n"
|
|
|
|
# Now, the enum names
|
|
print "\ntypedef enum"
|
|
print "{"
|
|
for (tnm in types) {
|
|
split (tnm, indices, SUBSEP);
|
|
t = indices[1];
|
|
n = indices[2];
|
|
m = indices[3];
|
|
printf (" TUNABLE_ENUM_NAME(%s, %s, %s),\n", t, n, m);
|
|
}
|
|
print "} tunable_id_t;\n"
|
|
|
|
print "\n#ifdef TUNABLES_INTERNAL"
|
|
# Internal definitions.
|
|
print "# define TUNABLE_NAME_MAX " (max_name_len + 1)
|
|
print "# define TUNABLE_ALIAS_MAX " (max_alias_len + 1)
|
|
print "# include \"dl-tunable-types.h\""
|
|
# Finally, the tunable list.
|
|
print "static tunable_t tunable_list[] attribute_relro = {"
|
|
for (tnm in types) {
|
|
split (tnm, indices, SUBSEP);
|
|
t = indices[1];
|
|
n = indices[2];
|
|
m = indices[3];
|
|
printf (" {TUNABLE_NAME_S(%s, %s, %s)", t, n, m)
|
|
printf (", {TUNABLE_TYPE_%s, %s, %s}, {%s}, NULL, TUNABLE_SECLEVEL_%s, %s},\n",
|
|
types[t,n,m], minvals[t,n,m], maxvals[t,n,m],
|
|
default_val[t,n,m], security_level[t,n,m], env_alias[t,n,m]);
|
|
}
|
|
print "};"
|
|
print "#endif"
|
|
}
|