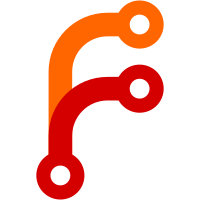
2004-10-27 Derek R. Price <derek@ximbiot.com> [BZ #487] This change is imported from gnulib. * time/mktime.c (not_equal_tm) [DEBUG]: Remove redundant check. 2004-10-24 Paul Eggert <eggert@cs.ucla.edu> [BZ #473] * time/tst-mktime.c (main): Don't assume that mktime fails when given time stamps before 1970. It returns negative time_t values instead, for compatibility with BSD. * time/tst-mktime2.c: New file. * time/Makefile (tests): Add it. [BZ #473] Import from gnulib. Revamp to avoid several problems near time_t extrema, and on hosts with 64-bit time_t and 32-bit int. This fixes Debian bug 177940. * time/mktime.c (TIME_T_MIDPOINT): New macro. (ydhms_diff): Renamed from ydhms_tm_diff, with a new signature, which avoids overflow problems on hosts with 64-bit time_t and 32-bit int. All callers changed. Now an inline function. Verify at compile-time that long int is wide enough to avoid these overflow problems. (guess_time_tm): New function. (__mktime_internal): Use it. Avoid overflow when computing yday on hosts with 64-bit long and 32-bit int. Remove tests for 69; no longer needed. Use if rather than #ifdef for LEAP_SECONDS_POSSIBLE so that the code is checked by more compilers. Do not rely on floating point to probe: stick to integer arithmetic, to avoid potential porting problems. Repair potential overflow correctly in the Southern Hemisphere. (localtime_offset): Add a FIXME for the case where time_t is unsigned.
71 lines
1.4 KiB
C
71 lines
1.4 KiB
C
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <time.h>
|
|
|
|
int
|
|
main (void)
|
|
{
|
|
struct tm time_str, *tm;
|
|
time_t t;
|
|
char daybuf[20];
|
|
int result;
|
|
|
|
time_str.tm_year = 2001 - 1900;
|
|
time_str.tm_mon = 7 - 1;
|
|
time_str.tm_mday = 4;
|
|
time_str.tm_hour = 0;
|
|
time_str.tm_min = 0;
|
|
time_str.tm_sec = 1;
|
|
time_str.tm_isdst = -1;
|
|
|
|
if (mktime (&time_str) == -1)
|
|
{
|
|
(void) puts ("-unknown-");
|
|
result = 1;
|
|
}
|
|
else
|
|
{
|
|
(void) strftime (daybuf, sizeof (daybuf), "%A", &time_str);
|
|
(void) puts (daybuf);
|
|
result = strcmp (daybuf, "Wednesday") != 0;
|
|
}
|
|
|
|
setenv ("TZ", "EST+5", 1);
|
|
#define EVENING69 1 * 60 * 60 + 2 * 60 + 29
|
|
t = EVENING69;
|
|
tm = localtime (&t);
|
|
if (tm == NULL)
|
|
{
|
|
(void) puts ("localtime returned NULL");
|
|
result = 1;
|
|
}
|
|
else
|
|
{
|
|
time_str = *tm;
|
|
t = mktime (&time_str);
|
|
if (t != EVENING69)
|
|
{
|
|
printf ("mktime returned %ld, expected %d\n",
|
|
(long) t, EVENING69);
|
|
result = 1;
|
|
}
|
|
else
|
|
(void) puts ("Dec 31 1969 EST test passed");
|
|
|
|
setenv ("TZ", "CET-1", 1);
|
|
t = mktime (&time_str);
|
|
#define EVENING69_CET (EVENING69 - (5 - -1) * 60 * 60)
|
|
if (t != EVENING69_CET)
|
|
{
|
|
printf ("mktime returned %ld, expected %ld\n",
|
|
(long) t, (long) EVENING69_CET);
|
|
result = 1;
|
|
}
|
|
else
|
|
(void) puts ("Dec 31 1969 CET test passed");
|
|
}
|
|
|
|
return result;
|
|
}
|