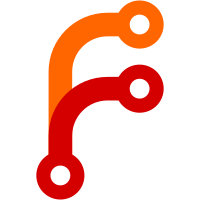
* pwd/fgetpwent.c: Don't include ../nss/nss_files/files-parse.c to define parse_line function. (parse_line): #define to _nss_files_parse_pwent and add extern decl for that. * nss/nss_files/files-XXX.c (internal_getent): Return NSS_STATUS_TRYAGAIN for ERANGE error. * sysdeps/i386/strtok.S (LreturnNULL): Save current state ptr instead of null, so next round returns null again instead of bombing. Fix from drepper. * nss/nss_files/files-parse.c (LINE_PARSER): Take new first arg EOLSET. Remove ; after `ENTDATA_DECL (data)'. Truncate line at strpbrk (line, EOLSET "\n"). (ENTDATA_DECL): Put ; at end. (MIDLINE_COMMENTS): Macro removed. * nss/nss_files/files-ethers.c: Pass new argument. * nss/nss_files/files-hosts.c: Likewise. * nss/nss_files/files-network.c: Likewise. * nss/nss_files/files-parse.c: Likewise. * nss/nss_files/files-proto.c: Likewise. * nss/nss_files/files-rpc.c: Likewise. * nss/nss_files/files-service.c: Likewise. * grp/fgetgrent.c: Likewise. * pwd/fgetpwent.c: Likewise. * nss/nss_files/files-pwd.c: Get parse_line with extern decl, since fgetpwent.c already defines it. * nss/nss_files/files-grp.c: Likewise. * elf/dl-load.c (_dl_map_object): Use any object with matching l_name as well as any matching with l_libname. Fix DT_SONAME lookup to use string table properly. * elf/rtld.c (dl_main): Set _dl_rtld_map.l_name from _dl_argv[0] when invoked directly, and l_libname from that if PT_INTERP missing. Set l_name from l_libname only if not set from argv. * time/europe, time/northamerica: Updated from ADO 96i. * stdio-common/tst-ungetc.c: Include unistd.h.
64 lines
1.9 KiB
C
64 lines
1.9 KiB
C
/* Copyright (C) 1991, 1996 Free Software Foundation, Inc.
|
|
This file is part of the GNU C Library.
|
|
|
|
The GNU C Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The GNU C Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the GNU C Library; see the file COPYING.LIB. If
|
|
not, write to the Free Software Foundation, Inc., 675 Mass Ave,
|
|
Cambridge, MA 02139, USA. */
|
|
|
|
#include <stdio.h>
|
|
#include <grp.h>
|
|
|
|
/* Define a line parsing function using the common code
|
|
used in the nss_files module. */
|
|
|
|
#define STRUCTURE group
|
|
#define ENTNAME grent
|
|
struct grent_data {};
|
|
|
|
#define TRAILING_LIST_MEMBER gr_mem
|
|
#define TRAILING_LIST_SEPARATOR_P(c) ((c) == ',')
|
|
#include "../nss/nss_files/files-parse.c"
|
|
LINE_PARSER
|
|
(,
|
|
STRING_FIELD (result->gr_name, ISCOLON, 0);
|
|
STRING_FIELD (result->gr_passwd, ISCOLON, 0);
|
|
INT_FIELD (result->gr_gid, ISCOLON, 0, 10,);
|
|
)
|
|
|
|
|
|
/* Read one entry from the given stream. */
|
|
struct group *
|
|
fgetgrent (FILE *stream)
|
|
{
|
|
static char buffer[BUFSIZ];
|
|
static struct group result;
|
|
char *p;
|
|
|
|
do
|
|
{
|
|
p = fgets (buffer, sizeof buffer, stream);
|
|
if (p == NULL)
|
|
return NULL;
|
|
|
|
/* Skip leading blanks. */
|
|
while (isspace (*p))
|
|
++p;
|
|
} while (*p == '\0' || *p == '#' || /* Ignore empty and comment lines. */
|
|
/* Parse the line. If it is invalid, loop to
|
|
get the next line of the file to parse. */
|
|
! parse_line (p, &result, (void *) buffer, sizeof buffer));
|
|
|
|
return &result;
|
|
}
|