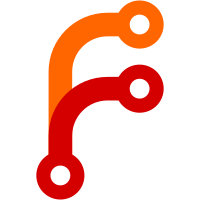
uthash's hashtable implementation will be used by libGLX for storing various mappings needed for correct dispatching.
35 lines
878 B
C
35 lines
878 B
C
#include <string.h> /* strcpy */
|
|
#include <stdlib.h> /* malloc */
|
|
#include <stdio.h> /* printf */
|
|
#include "uthash.h"
|
|
|
|
struct my_struct {
|
|
char name[10]; /* key */
|
|
int id;
|
|
UT_hash_handle hh; /* makes this structure hashable */
|
|
};
|
|
|
|
|
|
int main(int argc, char *argv[]) {
|
|
const char **n, *names[] = { "joe", "bob", "betty", NULL };
|
|
struct my_struct *s, *tmp, *users = NULL;
|
|
int i=0;
|
|
|
|
for (n = names; *n != NULL; n++) {
|
|
s = (struct my_struct*)malloc(sizeof(struct my_struct));
|
|
strncpy(s->name, *n,10);
|
|
s->id = i++;
|
|
HASH_ADD_STR( users, name, s );
|
|
}
|
|
|
|
HASH_FIND_STR( users, "betty", s);
|
|
if (s) printf("betty's id is %d\n", s->id);
|
|
|
|
/* free the hash table contents */
|
|
HASH_ITER(hh, users, s, tmp) {
|
|
HASH_DEL(users, s);
|
|
free(s);
|
|
}
|
|
return 0;
|
|
}
|