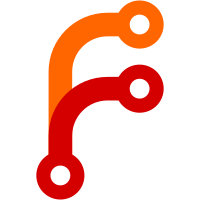
uthash's hashtable implementation will be used by libGLX for storing various mappings needed for correct dispatching.
29 lines
760 B
C
29 lines
760 B
C
#include <string.h> /* memcpy */
|
|
#include <stdlib.h> /* malloc */
|
|
#include <stdio.h> /* printf */
|
|
#include "uthash.h"
|
|
|
|
struct my_struct {
|
|
char bkey[5]; /* "binary" key */
|
|
int data;
|
|
UT_hash_handle hh;
|
|
};
|
|
|
|
int main(int argc, char *argv[]) {
|
|
struct my_struct *s, *t, *bins = NULL;
|
|
char binary[5] = {3,1,4,1,6};
|
|
|
|
/* allocate our structure. initialize to some values */
|
|
s = (struct my_struct*)calloc(1,sizeof(struct my_struct));
|
|
memcpy(s->bkey, binary, sizeof(binary));
|
|
|
|
/* add to hash table using general macro */
|
|
HASH_ADD( hh, bins, bkey, sizeof(binary), s);
|
|
|
|
/* look up the structure we just added */
|
|
HASH_FIND( hh, bins, binary, sizeof(binary), t );
|
|
|
|
if (t) printf("found\n");
|
|
return 0;
|
|
}
|