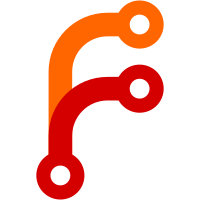
brackets, e.g. import <nixpkgs/pkgs/lib> are resolved by looking them up relative to the elements listed in the search path. This allows us to get rid of hacks like import "${builtins.getEnv "NIXPKGS_ALL"}/pkgs/lib" The search path can be specified through the ‘-I’ command-line flag and through the colon-separated ‘NIX_PATH’ environment variable, e.g., $ nix-build -I /etc/nixos ... If a file is not found in the search path, an error message is lazily thrown.
188 lines
4.7 KiB
Plaintext
188 lines
4.7 KiB
Plaintext
#! @perl@ -w -I@libexecdir@/nix
|
|
|
|
use strict;
|
|
|
|
my $binDir = $ENV{"NIX_BIN_DIR"} || "@bindir@";
|
|
|
|
|
|
my $addDrvLink = 0;
|
|
my $addOutLink = 1;
|
|
|
|
my $outLink;
|
|
my $drvLink;
|
|
|
|
my $dryRun = 0;
|
|
my $verbose = 0;
|
|
|
|
my @instArgs = ();
|
|
my @buildArgs = ();
|
|
my @exprs = ();
|
|
|
|
|
|
END {
|
|
foreach my $fn (glob ".nix-build-tmp-*") {
|
|
unlink $fn;
|
|
}
|
|
}
|
|
|
|
sub intHandler {
|
|
exit 1;
|
|
}
|
|
|
|
$SIG{'INT'} = 'intHandler';
|
|
|
|
|
|
for (my $n = 0; $n < scalar @ARGV; $n++) {
|
|
my $arg = $ARGV[$n];
|
|
|
|
if ($arg eq "--help") {
|
|
print STDERR <<EOF;
|
|
Usage: nix-build [OPTION]... [FILE]...
|
|
|
|
`nix-build' builds the given Nix expressions (which
|
|
default to ./default.nix if none are given). A symlink called
|
|
`result' is placed in the current directory.
|
|
|
|
Flags:
|
|
--add-drv-link: create a symlink `derivation' to the store derivation
|
|
--drv-link NAME: create symlink NAME instead of `derivation'
|
|
--no-out-link: do not create the `result' symlink
|
|
--out-link / -o NAME: create symlink NAME instead of `result'
|
|
--attr ATTR: select a specific attribution from the Nix expression
|
|
|
|
Any additional flags are passed to `nix-store'.
|
|
EOF
|
|
exit 0;
|
|
# '` hack
|
|
}
|
|
|
|
elsif ($arg eq "--add-drv-link") {
|
|
$addDrvLink = 1;
|
|
}
|
|
|
|
elsif ($arg eq "--no-out-link" or $arg eq "--no-link") {
|
|
$addOutLink = 0;
|
|
}
|
|
|
|
elsif ($arg eq "--drv-link") {
|
|
$n++;
|
|
die "$0: `$arg' requires an argument\n" unless $n < scalar @ARGV;
|
|
$drvLink = $ARGV[$n];
|
|
}
|
|
|
|
elsif ($arg eq "--out-link" or $arg eq "-o") {
|
|
$n++;
|
|
die "$0: `$arg' requires an argument\n" unless $n < scalar @ARGV;
|
|
$outLink = $ARGV[$n];
|
|
}
|
|
|
|
elsif ($arg eq "--attr" or $arg eq "-A" or $arg eq "-I") {
|
|
$n++;
|
|
die "$0: `$arg' requires an argument\n" unless $n < scalar @ARGV;
|
|
push @instArgs, ($arg, $ARGV[$n]);
|
|
}
|
|
|
|
elsif ($arg eq "--arg" || $arg eq "--argstr") {
|
|
die "$0: `$arg' requires two arguments\n" unless $n + 2 < scalar @ARGV;
|
|
push @instArgs, ($arg, $ARGV[$n + 1], $ARGV[$n + 2]);
|
|
$n += 2;
|
|
}
|
|
|
|
elsif ($arg eq "--log-type") {
|
|
$n++;
|
|
die "$0: `$arg' requires an argument\n" unless $n < scalar @ARGV;
|
|
push @instArgs, ($arg, $ARGV[$n]);
|
|
push @buildArgs, ($arg, $ARGV[$n]);
|
|
}
|
|
|
|
elsif ($arg eq "--option") {
|
|
die "$0: `$arg' requires two arguments\n" unless $n + 2 < scalar @ARGV;
|
|
push @instArgs, ($arg, $ARGV[$n + 1], $ARGV[$n + 2]);
|
|
push @buildArgs, ($arg, $ARGV[$n + 1], $ARGV[$n + 2]);
|
|
$n += 2;
|
|
}
|
|
|
|
elsif ($arg eq "--max-jobs" or $arg eq "-j" or $arg eq "--max-silent-time" or $arg eq "--log-type" or $arg eq "--cores") {
|
|
$n++;
|
|
die "$0: `$arg' requires an argument\n" unless $n < scalar @ARGV;
|
|
push @buildArgs, ($arg, $ARGV[$n]);
|
|
}
|
|
|
|
elsif ($arg eq "--dry-run") {
|
|
push @buildArgs, "--dry-run";
|
|
$dryRun = 1;
|
|
}
|
|
|
|
elsif ($arg eq "--show-trace") {
|
|
push @instArgs, $arg;
|
|
}
|
|
|
|
elsif ($arg eq "--verbose" or substr($arg, 0, 2) eq "-v") {
|
|
push @buildArgs, $arg;
|
|
push @instArgs, $arg;
|
|
$verbose = 1;
|
|
}
|
|
|
|
elsif ($arg eq "--quiet") {
|
|
push @buildArgs, $arg;
|
|
push @instArgs, $arg;
|
|
}
|
|
|
|
elsif (substr($arg, 0, 1) eq "-") {
|
|
push @buildArgs, $arg;
|
|
}
|
|
|
|
else {
|
|
push @exprs, $arg;
|
|
}
|
|
}
|
|
|
|
@exprs = ("./default.nix") if scalar @exprs == 0;
|
|
|
|
|
|
if (!defined $drvLink) {
|
|
$drvLink = "derivation";
|
|
$drvLink = ".nix-build-tmp-" . $drvLink if !$addDrvLink;
|
|
}
|
|
|
|
if (!defined $outLink) {
|
|
$outLink = "result";
|
|
$outLink = ".nix-build-tmp-" . $outLink if !$addOutLink;
|
|
}
|
|
|
|
|
|
foreach my $expr (@exprs) {
|
|
|
|
# Instantiate.
|
|
my @drvPaths;
|
|
# !!! would prefer the perl 5.8.0 pipe open feature here.
|
|
my $pid = open(DRVPATHS, "-|") || exec "$binDir/nix-instantiate", "--add-root", $drvLink, "--indirect", @instArgs, $expr;
|
|
while (<DRVPATHS>) {chomp; push @drvPaths, $_;}
|
|
if (!close DRVPATHS) {
|
|
die "nix-instantiate killed by signal " . ($? & 127) . "\n" if ($? & 127);
|
|
exit 1;
|
|
}
|
|
|
|
foreach my $drvPath (@drvPaths) {
|
|
my $target = readlink $drvPath or die "cannot read symlink `$drvPath'";
|
|
print STDERR "derivation is $target\n" if $verbose;
|
|
}
|
|
|
|
# Build.
|
|
my @outPaths;
|
|
$pid = open(OUTPATHS, "-|") || exec "$binDir/nix-store", "--add-root", $outLink, "--indirect", "-r",
|
|
@buildArgs, @drvPaths;
|
|
while (<OUTPATHS>) {chomp; push @outPaths, $_;}
|
|
if (!close OUTPATHS) {
|
|
die "nix-store killed by signal " . ($? & 127) . "\n" if ($? & 127);
|
|
exit 1;
|
|
}
|
|
|
|
next if $dryRun;
|
|
|
|
foreach my $outPath (@outPaths) {
|
|
my $target = readlink $outPath or die "cannot read symlink `$outPath'";
|
|
print "$target\n";
|
|
}
|
|
}
|