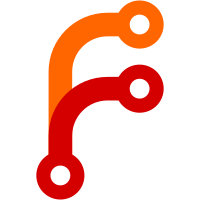
paths (e.g., `/nix/store/...random-hash...-aterm'), which are subsequently rewritten to actual content-addressable store paths (i.e., the hash part of the store path equals the hash of the contents). A complication is that the temporary output paths have to be passed to the builder (e.g., in $out). Likewise, other environment variables and command-line arguments cannot contain fixed store paths because their names are no longer known in advance. Therefore, we now put placeholder store paths in environment variables and command-line arguments, which we *rewrite* to the actual paths prior to running the builder. TODO: maintain the mapping of derivation placeholder outputs ("output path equivalence classes") to actual output paths in the database. Right now the first build succeeds and all its dependencies fail because they cannot find the output of the first. TODO: locking is no longer an issue with random temporary paths, but at the cost of having no blocking if we build the same thing twice in parallel. Maybe the "random" path should actually be a hash of the placeholder and the name of the user who started the build.
68 lines
1.6 KiB
C++
68 lines
1.6 KiB
C++
#ifndef __DERIVATIONS_H
|
|
#define __DERIVATIONS_H
|
|
|
|
#include "aterm.hh"
|
|
#include "store.hh"
|
|
|
|
|
|
/* Extension of derivations in the Nix store. */
|
|
const string drvExtension = ".drv";
|
|
|
|
|
|
/* Abstract syntax of derivations. */
|
|
|
|
struct DerivationOutput
|
|
{
|
|
OutputEqClass eqClass;
|
|
string hashAlgo; /* hash used for expected hash computation */
|
|
string hash; /* expected hash, may be null */
|
|
DerivationOutput()
|
|
{
|
|
}
|
|
DerivationOutput(OutputEqClass eqClass, string hashAlgo, string hash)
|
|
{
|
|
this->eqClass = eqClass;
|
|
this->hashAlgo = hashAlgo;
|
|
this->hash = hash;
|
|
}
|
|
};
|
|
|
|
typedef map<string, DerivationOutput> DerivationOutputs;
|
|
|
|
/* For inputs that are sub-derivations, we specify exactly which
|
|
output IDs we are interested in. */
|
|
typedef map<Path, StringSet> DerivationInputs;
|
|
|
|
typedef map<string, string> StringPairs;
|
|
|
|
struct Derivation
|
|
{
|
|
DerivationOutputs outputs; /* keyed on symbolic IDs */
|
|
DerivationInputs inputDrvs; /* inputs that are sub-derivations */
|
|
PathSet inputSrcs; /* inputs that are sources */
|
|
string platform;
|
|
Path builder;
|
|
Strings args;
|
|
StringPairs env;
|
|
};
|
|
|
|
|
|
/* Hash an aterm. */
|
|
Hash hashTerm(ATerm t);
|
|
|
|
/* Write a derivation to the Nix store, and return its path. */
|
|
Path writeDerivation(const Derivation & drv, const string & name);
|
|
|
|
/* Parse a derivation. */
|
|
Derivation parseDerivation(ATerm t);
|
|
|
|
/* Parse a derivation. */
|
|
ATerm unparseDerivation(const Derivation & drv);
|
|
|
|
/* Check whether a file name ends with the extensions for
|
|
derivations. */
|
|
bool isDerivation(const string & fileName);
|
|
|
|
|
|
#endif /* !__DERIVATIONS_H */
|